Steps
Declare a GridView control with a TemplateColumn as the
first column to include Radio control. Include BoundField for other fields to
display in the GridView.
<asp:GridView ID="gvUsers" runat="server"
AutoGenerateColumns="False" BackColor="White" BorderColor="#010101"
BorderStyle="Groove" BorderWidth="1px" CellPadding="4"
OnRowCommand="gvUsers_RowCommand">
<Columns>
<asp:TemplateField
HeaderText="Select">
<ItemTemplate>
<asp:RadioButton ID="rdbGVRow"
onclick="javascript:CheckOtherIsCheckedByGVID(this);" runat="server" />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Email"
HeaderText="Email" ReadOnly="True" />
<asp:BoundField DataField="FirstName"
HeaderText="First Name" ReadOnly="True" />
<asp:BoundField DataField="LastName"
HeaderText="Last Name" ReadOnly="True" />
</Columns>
<FooterStyle BackColor="White" ForeColor="#330099"
/>
<RowStyle BackColor="White" ForeColor="#330099"
/>
<SelectedRowStyle
BackColor="#FFCC66" Font-Bold="True" ForeColor="#663399" />
<PagerStyle BackColor="#FFFFCC" ForeColor="#330099"
HorizontalAlign="Center" />
<HeaderStyle BackColor="#F06300" Font-Bold="True"
ForeColor="#FFFFCC" />
</asp:GridView>
CodeBehind
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
BindUsers();
}
public void BindUsers()
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["Sql"].ConnectionString);
con.Open();
SqlCommand com = new
SqlCommand("SP_GetUsersForModeration", con);
com.CommandType = CommandType.StoredProcedure;
SqlDataAdapter ada = new SqlDataAdapter(com);
DataTable dt = new DataTable();
ada.Fill(dt);
gvUsers.DataSource = dt;
gvUsers.DataBind();
}
Execute the page. You can see the GridView with
RadioButton.
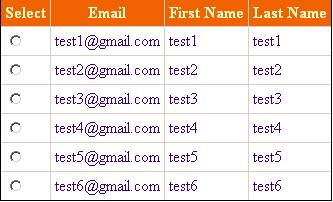
Adding Client Side Functionality
To check if there is some other row selected and to
uncheck it by keeping the current row selection we will call a JavaScript
function called CheckOtherIsCheckedByGVID(). This function is called by the OnClick event of the RadioButton, Refer the above code.
The below JavaScript function will do our client side functionality.
function CheckOtherIsCheckedByGVID(spanChk)
{
var IsChecked = spanChk.checked;
if(IsChecked)
{
spanChk.parentElement.parentElement.style.backgroundColor='#228b22';
spanChk.parentElement.parentElement.style.color='white';
}
var CurrentRdbID = spanChk.id;
var Chk = spanChk;
Parent =
document.getElementById('gvUsers');
var items =
Parent.getElementsByTagName('input');
for(i=0;i<items.length;i++)
{
if(items[i].id != CurrentRdbID &&
items[i].type=="radio")
{
if(items[i].checked)
{
items[i].checked = false;
items[i].parentElement.parentElement.style.backgroundColor='white';
items[i].parentElement.parentElement.style.color='black';
}
}
}
}
|