Getting Controls that use
__doPostBack() method
The controls that raises the postback
through __doPostBack() function fills 2 hidden variables defined by ASP.Net. One
of the hidden field __EVENTTARGET will be populated by the control ID of the
page elements that raised the postback.
Hence, in this case we can fetch the
control that raised the postback through the hidden field __EVENTTARGET.
Refer the below code,
public partial class _Default :
System.Web.UI.Page
{
protected void Page_Load(object
sender, EventArgs e)
{
if (IsPostBack)
{
string controlID =
Page.Request.Params["__EVENTTARGET"];
Control postbackControl =
Page.FindControl(controlID);
}
}
}
In the above code, if the postback
control is a LinkButton with ID LinkButton1 then the controlID will give you
LinkButton1.
Forcing Button to use __doPostBack()
method
There is a property called
UseSubmitBehaviour through which we can control the Button to use or not
to use __doPostBack() method. The default value of this property is true and
hence it uses browser’s ability to submit the form. When the property is set to
false, then the Button control also uses __doPostBack() method. Hence, the below
code is valid to fetch the Button control if it raises the postback.
ASPX
<asp:Button ID="Button1" runat="server"
Text="Button"
UseSubmitBehavior="False"
/>
CodeBehind
public partial class _Default :
System.Web.UI.Page
{
protected void Page_Load(object
sender, EventArgs e)
{
if (IsPostBack)
{
string controlID =
Page.Request.Params["__EVENTTARGET"];
Control postbackControl =
Page.FindControl(controlID);
}
}
}
Button and ImageButton
control
The technique discussed in the previous
section will not work for ImageButton control and a Button control by default.
The other way to find a Button control or an ImageButton control is by looping
the posted Request.Form collection and finding the control. One good thing that
can make our life easy is, when there is multiple Button or ImageButton controls
present in the form then the particular Button control or ImageButton which
raised the postback will alone be posted to the server through the form
collection and rest of the Button controls will be ignored. In other words, if
there is multiple Button or ImageButton control that can raise postback in form
then the Request.Form collection will include only one Button or ImageButton
control that raised the postback.
In the Request.Form collection, if the
postback element is Button control then there will be one entry for the Button
control with the ID of the control. If it is an ImageButton then there will be 2
entries with ImageButtonId suffixed by .x and .y to indicate the mouse pointer
location through x and y coordinates on the image when it is clicked. Look at
the below visual studio QuickWatch window when an ImageButton with ID
ImageButton1 is clicked.
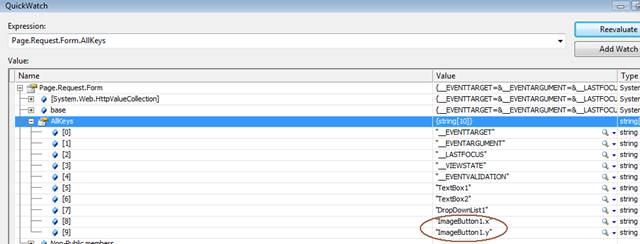
The below QuickWatch window shows a
Button control with ID Button1.
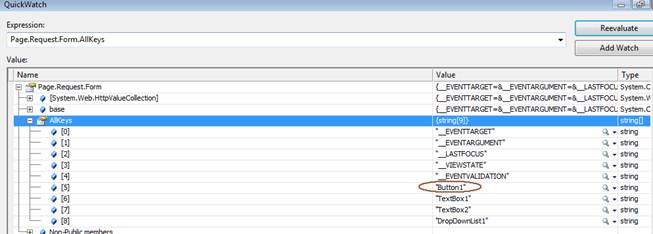
Thus, if
Page.Request.Params["__EVENTTARGET"] does not succeeds then find a Button or
ImageButton control from the Request.Form collection.
Refer the below code,
protected void Page_Load(object sender,
EventArgs e)
{
if (IsPostBack)
{
string controlID =
Page.Request.Params["__EVENTTARGET"];
Control postbackControl =
null;
if (controlID != null
&& controlID != String.Empty)
{
postbackControl =
Page.FindControl(controlID);
}
else
{
foreach (string ctrl in
Page.Request.Form)
{ //Check if Image
Button
if
(ctrl.EndsWith(".x") || ctrl.EndsWith(".y"))
{
postbackControl =
Page.FindControl(ctrl.Substring(0, ctrl.Length - 2));
break;
}
else
{
postbackControl =
Page.FindControl(ctrl);
//Check if Button
control
if (postbackControl is
Button)
{
break;
}
}
}
}
Response.Write(postbackControl.ID);
}
}
|