Moving forward, we will see how to develop a feature
which can provide the capability to upload multiple files without having any
restriction on number of files. In order to this, we develop a feature where
user can add as many as FileUpload control by clicking a link called “Add
file..”.
Refer the below figure,
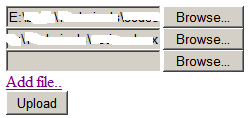
To add more file upload control, user has to click “Add
file..” link which will add another control to the control list. We will use
jQuery library to do the client side interaction in this article.
Doing Multiple File Uploads in ASP.Net
Steps
1.
Create a new Asp.Net project using your Visual Studio 2008 IDE with the language of your choice.
2. If you are new to jquery, read the following
Faq’s to integrate jQuery library into your project.
What is
jQuery? How to use it in ASP.Net Pages?
How to
enable jQuery intellisense in Visual Studio 2008?
How to use
jQuery intellisense in an external javascript file?
3. Create a folder in server and
provide appropriate permission (Read, Write & Modify)to the ASP.Net service
account in order to save the uploaded files. Configure this folder in
AppSettings of the project so that it can be accessed from code.
4. Drag a Button control from
visual studio toolbox to upload the files to server. Also, drag a Label control
to display success/failure message.
5. Now, with the help of jQuery
library we will provide a way to add as many file upload control in the page.
Refer the code below,
ASPX
<script type="text/javascript"
src="_scripts/jquery-1.4.1.min.js"></script>
<script type="text/javascript">
var i = 1;
$(document).ready(function() {
$("#addfile").click(function() {
$("#dvfiles").append("<input
name="+i+"fu type=file /><br>");
i++;
});
});
</script>
</head>
<body>
<form id="form1" runat="server"
enctype="multipart/form-data">
<div id="dvfiles">
</div>
<a href="#" id="addfile">Add file..</a><br
/>
<asp:Label ID="lblMessage"
runat="server"></asp:Label><br />
<asp:Button ID="btnUpload" runat="server" Text="Upload"
onclick="btnUpload_Click" />
</form>
Codebehind
protected void btnUpload_Click(object sender, EventArgs
e)
{
try
{
HttpFileCollection filecolln =
Request.Files;
for (int i = 0; i < filecolln.Count;
i++)
{
HttpPostedFile file = filecolln[i];
if (file.ContentLength > 0)
{
file.SaveAs(ConfigurationManager.AppSettings["FilePath"] +
System.IO.Path.GetFileName(file.FileName));
}
}
lblMessage.Text = "Uploaded Successfully!";
}
catch (Exception ex)
{
lblMessage.Text = ex.Message;
}
}
The above code will populate the file upload control in
the div tag (dvfiles) whenever addfile hyperlink is clicked.
Note
Please note the enctype attribute in the form tag
above. If you use ASP.Net FileUpload control, this attribute will be populated
automatically in form tag. But, here we need to specify this attribute
explicitly since we are populating the file upload (HTML file control) controls
ourselves from client side. Without setting this attribute, the file upload will
not work.
6. It's done! Execute and
see it in action.
|