If we consider the above scenario to use an ASPX page to
fulfill a single database operation where the other page events are not
necessary i.e. the ASPX page will have a series of event starting from Init to
Unload which is an overhead to this simple database operation. One can
understand the complexity if we are trying to populate more images in a single
page using the above approach. It is in this place we can think about using
HttpHandler to do the above process. As we know the HttpHandler will be the
endpoint for the request as opposed to the above ASPX page. Now the HttpHandler
will have only one method ProcessRequest which does the simple operation and
thus preventing a series of events. So how does the ProcessRequest method get
the access of Response object? If we see the signature of ProcessRequest it will
be like,
public void ProcessRequest (HttpContext context)
{
}
The context object will give access to necessary object about
the request. It is through which we can do a BinaryWrite using Response object.
Other scenarios where we can use HttpHandler is, if we want to export the
dataset content to Excel and if we want to have a file with our own extensions
like .axd we can use HttpHandler. We have to make appropriate config setting in
Web.Config to work,
<httpHandlers>
<add verb="supported http verbs" path="path"
type="namespace.classname, assemblyname" />
<httpHandlers>
Where verb are “GET, POST”, path will be like “*.axd” for the
above said example and type will be the actual type of the handler.
Developing a HttpHandler using Visual Studio 2005
Create a new WebApplication project. Right click the solution
and click “Add New Item” menu which will open the new items dialog box like,
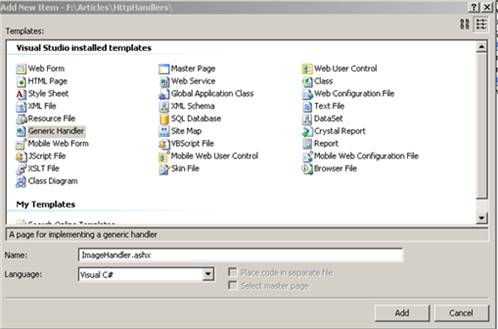
Select Generic handler and click Add. Now add the following
code,
<%@ WebHandler Language="C#" Class="ImageHandler" %>
using System;
using System.Web;
using System.Configuration;
using System.Data.SqlClient;
public class ImageHandler : IHttpHandler {
public void ProcessRequest (HttpContext context) {
string imageid =
context.Request.QueryString["ImID"];
SqlConnection connection = new
SqlConnection(ConfigurationManager.ConnectionStrings["connectionString"].ConnectionString);
connection.Open();
SqlCommand command = new SqlCommand("select Image
from Image where ImageID="+imageid, connection);
SqlDataReader dr = command.ExecuteReader();
dr.Read();
context.Response.BinaryWrite((Byte[])dr[0]);
connection.Close();
context.Response.End();
}
public bool IsReusable {
get {
return false;
}
}
}
To display the image in ASPX page,
imImage.ImageUrl = "~/ImageHandler.ashx?ImID=100;
Where imImage is image control and ImageHandler.ashx is the
HttpHandler.
|