For long time, there are no inbuilt
chart controls in the .NetFramework that can be used in these scenarios. Most of
the time, we will either use a 3rd party chart control or write our
own chart control to impress the business users. Both these approach has its own
drawbacks, one involves additional cost and other involves additional effort
(again, more cost!!).
But, this difficulty was no more since
Microsoft released their own free chart controls to use from .NetFramework 3.5
sp1. These chart controls comes as a separate download. This means, you need to
download and install these components manually if you require it. This chart
controls are visually stunning and feature rich. There are around 35 different
chart types like bar, graph, pie charts etc which can be used in both windows
and web based application.
Moving forward, let’s see how to use
this chart control in ASP.Net application.
Pre-Requisites
As is said earlier, the Microsoft chart
controls comes a separate download which needs to be downloaded and installed.
There are 2 installers that are required for this, one is the actual chart
controls assembly and the other is the add-on for your visual studio 2008 to
support the development environment.
Microsoft Chart Controls for
Microsoft .NET Framework 3.5
Microsoft Chart Controls Add-on for
Microsoft Visual Studio 2008
Another pre-requisite is, the above
component will require .NetFramework sp1, make sure you have this before
installing the above components.
Once you install the component, it will
add a new assembly called System.Web.DataVisualization.dll in your GAC. This has
all the related class to create charts in your application.
Once installed, the chart controls
should be part of “Data” tab of visual studio toolbox. If you didn’t find it
there, then you can add it to your toolbox by right clicking on the
toolbox>Choose items..> Select “Chart” under
“System.Web.DataVisualization.Charting” namespace in .NetFramework component
> then click OK.
With this information and assuming you
have installed the above installers, we will move ahead and understand how to
use the chart controls in ASP.Net applications using visual studio
2008.
Using Microsoft Free Chart control in
ASP.Net
Steps
1. You can create a new Asp.Net website project in your Visual Studio 2008 using the language of your choice.
In this article, i have used C# as the language of my choice.
2. To understand this article, we will use a simple database that has
Employee and Department table in App_Data folder. See below,
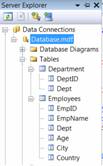
Using above
database; we will display number of employees against each department using
chart control.
3. Drag and drop a chart control into your aspx page. The code should
look like below,
<asp:Chart ID="Chart1"
runat="server">
<Series>
<asp:Series
Name="Series1">
</asp:Series>
</Series>
<ChartAreas>
<asp:ChartArea
Name="ChartArea1">
</asp:ChartArea>
</ChartAreas>
</asp:Chart>
If you look at the above code, it is
required that you need to configure a Series in Series collection and a
ChartArea in ChartAreas collection at a minimum to generate the
chart.
Before diving into this, let’s first
fetch the number of employees in each department. This can be done with either a
DataSource control or by writing your own ADO.Net code. Let’s see both in this
article, but for now we will stick to SqlDataSource control.
4. Drag a SqlDataSource control from the “Data” tab of Visual Studio
toolbar. Configure the SqlDataSource control through “Configure Data Source...”
wizard to fetch the data from the Sql express database added into your solution.
In “Configure the Select statement” step select “Specify a custom SQL statement
or stored procedure” and provide the below query,
SELECT COUNT(e.EmpID) AS EmpCount, d.Dept
FROM Employees AS e INNER JOIN Department AS d ON e.Dept = d.DeptID
GROUP BY d.Dept
The final control will look
like,
<asp:SqlDataSource ID="SqlDataSource1"
runat="server"
ConnectionString="<%$
ConnectionStrings:ConnectionString %>" SelectCommand="SELECT COUNT(e.EmpID)
AS EmpCount, d.Dept
FROM Employees AS e INNER
JOIN
Department AS d ON
e.Dept = d.DeptID
GROUP BY
d.Dept"></asp:SqlDataSource>
5. Set the DataSourceID of the Chart control to ID of our configured
SqlDataSource control. To do this, select the smart tag of the chart control in
the design viewer and configure “Choose Data Source:” property.
6. Now, it’s time to configure the Series and ChartAreas collection.
This can be done in 2 ways, by directly typing the code in
<Series></Series> and <ChartAreas></ChartAreas> or by
using visual studio property window. We will use the second approach in this
article since it is easy and we are yet to know the properties it supports. It’s
good to know, what Series and ChartArea is about before configuring
it.
Series are chart elements that store a collection of related data points and
also have an associated chart type (i.e. chart types are specified on a
series basis). It is here we indicate the column name to pickup for X
axis and Y axis data to draw the chart.
ChartArea are chart components that are used to plot a chart's data. Here, we
can specify the entire configurations related to the charts like, axis
title, appearance, etc. Hence, a series can be associated to ChartArea. A
combination of Series and ChartArea will help the chart control to draw the
chart using coordinates defined in Series and appearance of plot area
defined in ChartArea.
A
Chart control can have an unlimited number of ChartArea objects. Chart areas can
also be overlayed on top of one another.
Now,
let’s configure these 2 collections. Go to property window of the Chart control
and click the “...” button in Series property. This will open a dialog that
allows you to add new Series object and configure it. By default, when it opens
it will display a Series object. Select your chart type in ChartType
property; by default it will use “Column”. Scroll to Data Source section and
configure XValueMember as Dept and YValueMember to EmpCount. Click
OK.
To
configure ChartArea, click the “...” button in ChartAreas property. This will
open the dialog similar to previous step. Again click the “...” button in
Axes collection property. Select X axis and provide a title
“Department”. Select Y axis and provide the title as “Number of Employees”.
Click OK > OK. This will generate the below code in your aspx
page.
<asp:Chart ID="Chart1" runat="server"
DataSourceID="SqlDataSource1"
Height="600px" Palette="None"
Width="600px">
<series>
<asp:Series Name="Series1"
XValueMember="Dept"
YValueMembers="EmpCount"
ChartArea="ChartArea1" ChartType="Column" >
</asp:Series>
</series>
<chartareas>
<asp:ChartArea
Name="ChartArea1">
<AxisY Title="Number of
Employees">
</AxisY>
<AxisX
Title="Department">
</AxisX>
</asp:ChartArea>
</chartareas>
</asp:Chart>
7. That’s it. Execute your application and see it in action. Below is
the output generated by the chart control for our data.
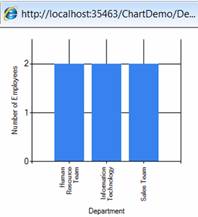
Once executed, the chart control will
basically render a PNG image in IMG tag with the chart image like
below,
<img id="Chart1"
src="/ChartDemo/ChartImg.axd?i=chart_f12224054e15413b9ad73d54dfd901b6_1.png &g=9f88a9e3882f4fdf9ad042ff6e61de74"
alt="" style="height:300px;width:300px;border-width:0px;" />
Enabling 3D chart
To enable 3D charts, go to ChartArea
Collection editor [click the “...” button in ChartAreas property]. Set (Enable
3D) to true under Area3DStyle. Refer below image,
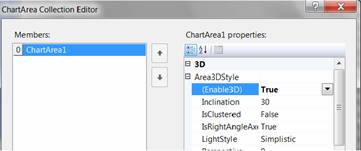
That’s it. Execute and see it in
action.
|