Also, you cannot create a derived class if the class is sealed.
In this case, we have to use extension method to add a new method.
You can create an extension method for
a class or interface in C#.
How to declare an extension
method?
An extension method should be a static
method in a static class. The first argument should be the type to which we are
adding the extension method with this modifier.
To demonstrate usage of extension
method, we will try to add a extension method called StripHTMLTags() to String
class which will strip the html tags contained in the string.
using System;
using
System.Text.RegularExpressions;
namespace ExtensionDemo
{
public static class
StringExtension
{
public static string StripHTMLTags(this
string str)
{
string expn = "<.*?>";
return Regex.Replace(str, expn,
string.Empty);
}
}
}
As I said before, once an extension
method is created for a type it can be accessed like a normal instance method.
Also, you can see the extension method appearing in the visual studio
intellisense for the string type henceforth. Refer the code below,
protected void Page_Load(object sender,
EventArgs e)
{
string HTML = "<b>I Love
ASP.Net!!</b>";
Response.Write(HTML.StripHTMLTags());
}
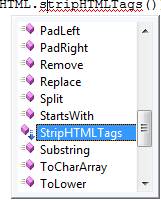
Scope of extension method
To call the extension method one has to
import the namespace through using directive where the extension method is
defined i.e. you will able to call or visual studio intellisense will show your
extension method only when you import the namespace where you have defined your
extension method. Refer the code below,
using System;
using
System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using
System.Web.UI.WebControls;
using ExtensionDemo;
public partial class _Default :
System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{
string HTML = "<b>I Love
ASP.Net!!</b>";
Response.Write(HTML.StripHTMLTags());
}
}
If the above code did not contain
“using ExtensionDemo;” then it will throw a compilation error (even intellisense
will not show the method). Once executed, the above code will produce output
without html tags.
OUTPUT
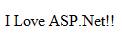
If you create an extension method with
similar signature of any methods contained in the type, then your extension
method will not be called. In this case, compiler will always give priority to
the instance method wherever it is called.
using System;
using
System.Text.RegularExpressions;
/// <summary>
/// Summary description for
StringExtension
/// </summary>
namespace ExtensionDemo
{
public static class
StringExtension
{
public static string StripHTMLTags(this
string str)
{
string expn = "<.*?>";
return Regex.Replace(str, expn,
string.Empty);
}
public static string ToString(this string
str)
{
string expn = "<.*?>";
return Regex.Replace(str, expn,
string.Empty);
}
}
}
In the above code, the second method
ToString() has similar signature of ToString() in String class. Hence, calling
ToString() on the string instance will always call the original implementation
and not the extension method. If you execute the below code, the output will
contain the html tags in the string variable HTML.
|