This article deals with the development of a little game
called “Paddling Ball” using WPF technology and is just a demo-like game that
uses minor user interactions with not so much of graphical items in it.
Game Window
XAML allows you to create menu, ball and pad objects
declaratively as below:
<Window x:Class="PaddingBall.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Padding Ball
v1.0" Height="500"
Width="700" Background="Gray" Name="playground"
ResizeMode="NoResize"
WindowStartupLocation="CenterScreen"
SizeToContent="Manual">
<Canvas
Width="700" Height="500">
<Menu
VerticalAlignment="Top"
HorizontalAlignment="Left"
Height="20"
Width="700" Background="AliceBlue" Foreground="Blue">
<MenuItem
Header="File">
<MenuItem Header="Start Game"
Background="AliceBlue"
Click="StartGame"></MenuItem>
<MenuItem
Header="Exit" Background="AliceBlue" Click="ExitGame"></MenuItem>
</MenuItem>
<MenuItem
Header="About"
Click="ShowAboutBox"></MenuItem>
</Menu>
<Grid
Height="462" Width="700">
<Grid.ColumnDefinitions>
<ColumnDefinition
Width="700*" />
<ColumnDefinition
Width="0*" />
<ColumnDefinition
Width="0*" />
</Grid.ColumnDefinitions>
<Rectangle
Margin="114,132,0,0" Name="ball" Stroke="Black"
RadiusX="120" RadiusY="120" Fill="Blue"
Height="38" VerticalAlignment="Top" Stretch="UniformToFill"
HorizontalAlignment="Left"
Width="38">
<Rectangle.BitmapEffect>
<BevelBitmapEffect BevelWidth="11" />
</Rectangle.BitmapEffect>
<Rectangle.BitmapEffectInput>
<BitmapEffectInput />
</Rectangle.BitmapEffectInput>
</Rectangle>
<Rectangle
Height="13" Margin="200,390,0,0" Name="pad"
Stroke="Black"
VerticalAlignment="Bottom"
Fill="Black"
HorizontalAlignment="Left" Width="100"
/>
</Grid>
</Canvas>
</Window>
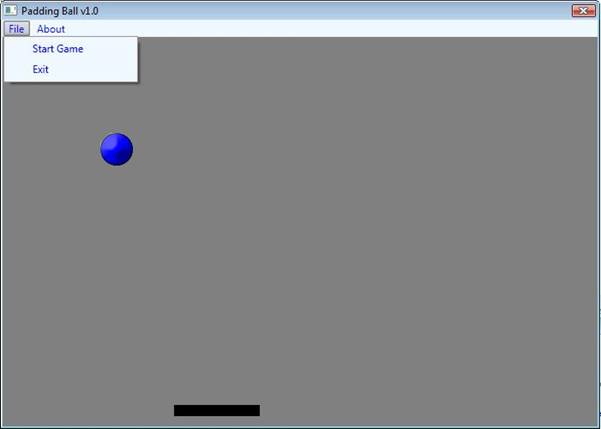
As you look at it, each of the menuitem is bind to the
respective event handlers for the Click event. When you open the application,
you could see a window with the Menu bar on top of it. You start the game by
selecting the “Start Game” command in the File menu.
WPF implications
While developing this game with WPF, I had experienced a
part easier when the drawings for Ball and Pad, the background are created,
thanks to XAML. On the other hand, the behaviour part of the game still had its
own intricacies especially during the animation of the ball and pad.
Nevertheless, the controlling of animation with the keyboard interaction by the
user had also thrown new ideas in WPF programming.
Most of the animation and positioning of ball and pad
was done with the Thickness structure found in System.Windows
namespace and ThicknessAnimation class found in
System.Windows.Media.Animation namespace of the
PresentationFramework.dll assembly.
The Thickness structure describes the thickness of a
frame around a rectangle, in this case, Ball and Pad. Four Double values
describe the Left, Top, Right, and Bottom sides of the rectangle that
encapsulates the Ball and Pad objects, respectively.
The ThicknessAnimation class creates a transition
between two target values. To set its target values, use its From and To
properties of the Ball and Pad elements inside the window.
Needless to say, we need a StoryBoard object to host the
whole animation. The ThicknessAnimation instances created for Ball and the Pad
objects, along with the specified duration is added as a child to the StoryBoard
and use the Begin method to start the animation.
Here is the code for animating the Pad as the user
presses the Right and Left arrow keys.
|