Now, build the project. If there is no build error, it
means that you have successfully created your first WCF service. If you see a
compilation error, such as "'ServiceModel' does not exist in the namespace
'System'", this is probably because you didn't add the ServiceModel
namespace reference correctly. Revisit the previous section to add this
reference, and you are all set.
Next, we will host this WCF service in an environment
and create a client application to consume it.
Hosting the WCF service in ASP.NET Development Server
The HelloWorldService is a class library. It has
to be hosted in an environment so that client applications may access it. In
this section, we will explain how to host it using the ASP.NET Development
Server.
Creating the host application
There are several built-in host applications for WCF
services within Visual Studio 2008. However, in this section, we will manually
create the host application so that you can have a better understanding of what
a hosting application is really like under the hood.
To host the library using the ASP.NET Development
Server, we need to add a new web site to the solution. Follow these steps to
create this web site:
1. In the Solution Explorer,
right-click on the solution file, and select Add | New Web Site…
from the context menu. The Add New Web Site dialog window should pop
up.
2. Select Empty Web Site as
the template, and leave the Location set as File System, and
language as Visual C#. Change the web site name from WebSite1 to
HostDevServer, and click OK.
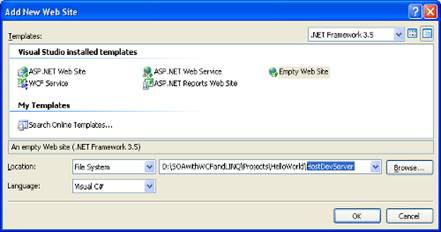
3. Now in the Solution Explorer,
you have one more item (HostDevServer) within the solution. It will look
like the following:
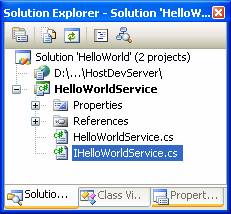
4. Next, we need to set the
website as the startup project. In the Solution Explorer, right-click on the web
site D:...HostDevServer, select Set as StartUp Project from the
context menu (or you can first select the web site from the Solution Explorer,
and then select menu item Website | Set as StartUp Project). The web site
D:...HostDevServer should be highlighted in the Solution Explorer
indicating that it is now the startup project.
5. Because we will host the
HelloWorldService from this web site, we need to add a
HelloWorldService reference to the web site. In the Solution Explorer,
right-click on the web site D:...HostDevServer, and select Add
Reference… from the context menu. The following Add Reference dialog
box should appear:
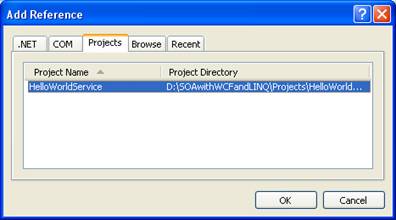
6. In the Add Reference
dialog box, click on the Projects tab, select HelloWorldService
project, and then click OK. You will see that a new directory (bin) has
been created under the HostDevServer web site, and two files from
HelloWorldService project have been copied to this new directory. Later
on, when this web site is accessed, the web server (either ASP.NET Development
Server or IIS) will look for executable code in this bin directory.
Testing the host application
Now we can run the website inside the ASP.NET
Development Server. If you start the web site HostDevServer, by pressing
Ctrl+F5, or select the Debug | Start Without Debugging… menu, you
will see an empty web site in your browser. Because we have set this website as
the startup project, but haven't set any start page, it lists all of the files
and directories inside the HostDevServer directory (Directory Browsing is
always enabled for a website within the ASP.NET Development Server).
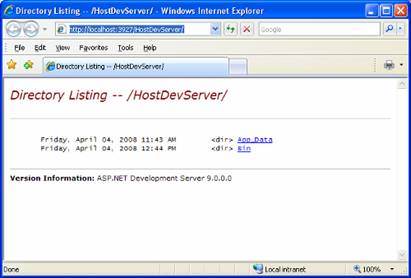
If you pressed F5 (or selected Debug | Start
Debugging from the menu), you may see a dialog saying Debugging Not
Enabled (as shown in the following figure). Choose the option Run without
debugging. (Equivalent to Ctrl+F5) and click the OK button to
continue. We will explore the debugging options of a WCF service later. Until
then, we will continue to use Ctrl+F5 to start the website without
debugging.
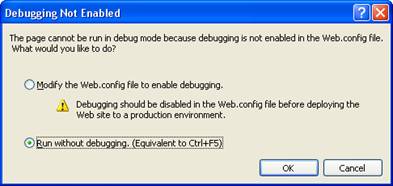
ASP.NET Development Server
At this point, you should have the HostDevServer
site up and running. This site is actually running inside the built-in ASP.NET
Development Server. It is a new feature that was introduced in Visual Studio
2005. This web server is intended to be used by developers only, and has
functionality similar to that of the Internet Information Services (IIS) server.
It also has some limitations; for example, you can run ASP.NET applications only
locally. You can't use it as a real IIS server to publish a web site.
By default, the ASP.NET Development Server uses a
dynamic port for the web server each time it is started. You can change it to
use a static port via the Properties page of the web site. Just change
the Use dynamic ports setting to false, and specify a static port, such
as 8080, from the Properties window of the HostDevServer web site.
You can't set the port to 80, because IIS is already using this port. However,
if you stop your local IIS, you can set your ASP.NET Development Server to use
port 80.
Even you set its port to 80 it is still a local web
server. It can't be accessed from outside your local PC.
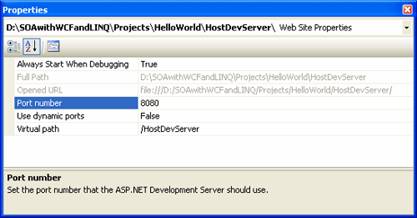
It is recommended that you use a static port so that
client applications know in advance where to connect to the service. From now
on, we will always use port 8080 in all of our examples.
The ASP.NET Development Server is normally started from
within Visual Studio when you need to debug or unit test a web project. If you
really need to start it from outside Visual Studio, you can use a command line
statement in the following format:
start /B WebDev.WebServer [/port:<port number>]
/path:<physical path> [/vpath:<virtual path>]
For our web site, the statement should be like this:
start /B webdev.webserver.exe /port:8080
/path:"D:SOAwithWCFandLINQ ProjectsHelloWorldHostDevServer"
/vpath:/HostDevServer
The webdev.webserver.exe is located under your
.NET framework installation directory
(C:WINDOWSMicrosoft.NETFrameworkv2.0.50727).
Adding an svc file to the host application
Although we can s tart the web site now, it is only an
empty site. Currently, it does not host our HelloWorldService. This is
because we haven't specified which service this web site should host, or an
entry point for this web site. Just as an asmx file is the entry point
for a non-WCF web service, a .svc file is the entry point for a WCF
service, if it is hosted on a web server. We will now add such a file to our web
site.
From the Solution Explorer, right-click on the web site
D:...HostDevServer, and select Add New Item… from the context
menu. The Add New Item dialog window should appear, as shown below.
Select Text File as the template, and change the Name from
TextFile.txt to HelloWorldService.svc in this dialog window.
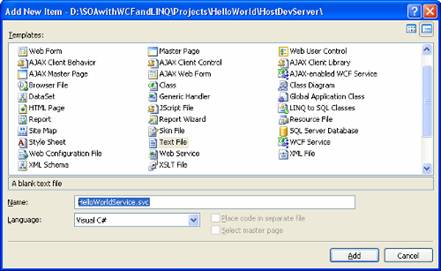
You may have noticed that there is a template, WCF
Service, in the list. We won't use it now as it will create a new WCF
service within this web site for you (we will use this template later).
After you click the Add button in the Add New
Item dialog box, an empty svc file will be created and added to the
web site. Now enter the following line in this file:
<%@ServiceHost
Service="MyWCFServices.HelloWorldService"%>
Adding a web.config file to the host application
The final step is to add a web.config file to the
web site. As in the previous step, add a text file named web.config to
the web site and enter the following code in this file:
<?xml version="1.0" encoding="utf-8"
?> <configuration> <appSettings> <add
key="HTTPBaseAddress"
value=""/> </appSettings> <system.serviceModel> <services> <service name="MyWCFServices.HelloWorldService" behaviorConfiguration="MyServiceTypeBehaviors"> <endpoint address="" binding="wsHttpBinding" contract="MyWCFServices.IHelloWorldService"/> <endpoint contract="IMetadataExchange" binding="mexHttpBinding" address="mex"
/> </service> </services> <behaviors> <serviceBehaviors> <behavior
name="MyServiceTypeBehaviors" > <serviceMetadata httpGetEnabled="true"
/> </behavior> </serviceBehaviors> </behaviors> </system.serviceModel> </configuration>
Within this file, we set HTTPBaseAddress to
empty, because this WCF service is hosted inside a web server and we will use
the web server default address as the service address.
The behavior httpGetEnabled is essential, because
we want other applications to be able to locate the metadata of this service.
Without the metadata, client applications can't generate the proxy and thus
won't be able to use the service.
We use wsHttpBinding for this hosting, which
means that it is secure (messages are encrypted while being transmitted), and
transaction-aware. However, because this is a WS-* standard, some existing
applications (for example: a QA tool) may not be able to consume this service.
In this case, you can change the service to use the basicHttpBinding,
which uses plain unencrypted texts when transmitting messages, and is backward
compatible with traditional ASP.NET web services (asmx web services).
The following is a brief explanation of the other
elements in this configuration file:
Ø
Configuration is the root node of the file.
Ø
Within the appSettings node, you can add
application-specific configurations. In this file, we have added one setting for
the base address of the web site.
Ø
system.serviceModel is the top node for all WCF service
specific settings.
Ø
Within the services node, you can specify WCF services that
are hosted on this web site. In our example, we have only one WCF service
HelloWorldService hosted in this web site.
Ø
Each service element defines one WCF service, including its
name, behavior, and endpoint.
Ø
Two endpoints have been defined for the HelloWorldService,
one for the service itself (an application endpoint), and another for the
metadata exchange (an infrastructure endpoint).
Ø
Within the serviceBehaviors node, you can define specific
behaviors for a service. In our example, we have specified one behavior, which
enables the service meta data exchange for the service.
Starting the host application
Now, if you start the web site by pressing
Ctrl+F5 (again, don't use F5 or menu option Debug | Start
Debugging until we discuss these, later), you will now find the file
HelloWorldService.svc listed on the web page. Clicking on this file will give
the description of this service, that is, how to get the wsdl file of
this service, and how to create a client to consume this service. You should see
a page similar to the following one. You can also set this file as the start
page file so that every time you start this web site, you will go to this page
directly. You can do this by right-clicking on this file in the Solution
Explorer and selecting Set as Start Page from the context menu.
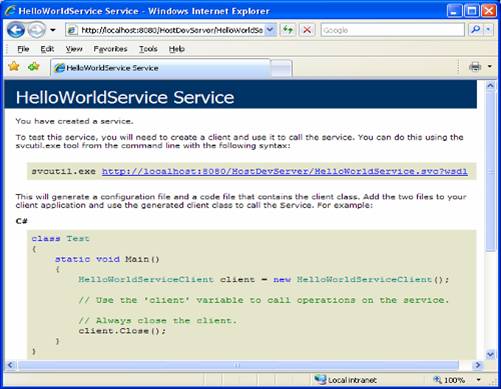
Now, click on the wsdl link on this page, and you
will get the wsdl xml file for this service. The wsdl file gives
all of the contract information for this service. In the next section, we will
use this wsdl to generate a proxy for our client application.
Close the browser. Then, from the Windows system tray
(systray), find the little icon labeled ASP.NET Development Server – Port
8080 (it is on the lower-right of your screen, just next to the clock),
right-click on it, and select Stop to stop the service.
Creating a client to consume the WCF service
Now that we have successfully created and hosted a WCF
service, we need a client to consume the service. We will create a C# client
application to consume the HelloWorldService.
In this section, we will create a Windows console
application to call the WCF service.
Creating the client application project
First, we need to create a console application project
and add it to the solution. Follow these steps to create the console
application:
1. In the Solution Explorer,
right-click on the solution HelloWorld, and select Add | New
Project… from the context menu. The Add New Project dialog window
should appear, as shown below.
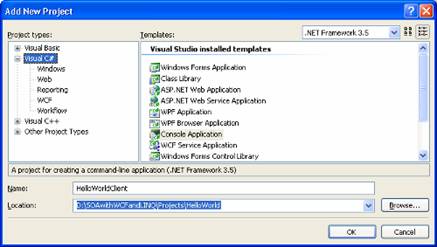
2. Select Visual C# Windows
as the project type, and Console Application as the template; change the
project name from the defaulted value of ConsoleApplication1 to
HelloWorldClient, and leave the location as
D:SOAwithWCFandLINQProjectsHelloWorld. Click the OK button. The
new client project has now been created and added to the solution.
Generating the proxy and configuration files
In order to consume a WCF service, a client application
must first obtain or generate a proxy class.
We also need a configuration file to specify things such
as the binding of the service, the address of the service, and the contract.
To generate these two files, we can use the
svcutil.exe tool from the command line. You can follow these steps to
generate the two files:
1. Start the service by pressing
Ctrl+F5 or by selecting menu option Debug | Start Without
Debugging (at this point your startup project should still be
HostDevServer; if not, you need to set this to be the startup project).
Now, you should see the window for the HelloWorldService service, as we
saw in the previous section.
2. After the service has been
started, run the command line svcutil.exe tool with the following
syntax:
"C:Program FilesMicrosoft
SDKsWindowsv6.0BinSvcUtil.exe" http://localhost:8080/HostDevServer/HelloWorldService.svc?wsdl /out:HelloWorldServiceRef.cs
/config:app.config
You will see output similar to that shown in the
following screenshot:
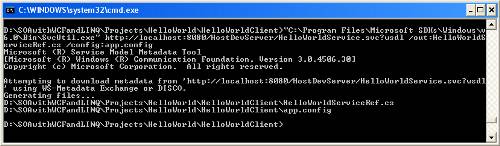
Now, two files have been generated— one for the proxy
(HelloWorldServiceRef.cs), and the other for the configuration
(app.config).
If you open the proxy file, you will see that the
interface of the service(IHelloWorldService) is mimicked inside the proxy
class, and a client class (HelloWorldServiceClient) is created to
implement this interface. Inside this client class, the implementation of the
service operation (GetMessage) is only a wrapper that delegates the call
to the actual service implementation of the operation.
Inside the configuration file, you will see the
definitions of the HelloWorldService, such as the endpoint address,
binding, timeout settings, and security behaviors of the service.
Customizing the client application
Before we can run the client application, we still have
some more work to do. Follow these steps to finish the customization:
1. Adding the two generated files
to the project: In the Solution Explorer, click Show All Files to show
all the files under the HelloWorldClient folder, and you will see these
two files. However, they are not included in the project. Right-click on each of
them and select Include In Project to include both of them in the client
project. You can also use menu Project | Add Existing Item … (or the
context menu Add | Existing Item …) to add them to the project.
2. Adding a reference to the
System.ServiceModel namespace: Just as we did for the project
HelloWorldService, we also need to add a reference to the WCF .NET
System.ServiceModel assembly. From the Solution Explorer, just
right-click on the HelloWorldClient project, select Add Reference…
and choose .NET System.ServiceModel. Then, click the OK button to
add the reference to the project.
3. Modify the program.cs to
call the service: In program.cs, add the following line to initialize the
service client object:
HelloWorldServiceClient client = new
HelloWorldServiceClient();
Then, we can call its method just as we would do for any
other object:
Console.WriteLine(client.GetMessage("Mike Liu"));
Pass your name as the parameter to the GetMessage
method, so that it prints out a message for you.
Running the client application
We are now ready to run this client program.
First, make sure the the HelloWorldService has
been started. If you previously stopped it, start it now.
Then, from the Solution Explorer, right-click on the
project HelloWorldClient, select Set as StartUp Project, and then
press Ctrl+F5 to run it.
You will see output as shown in the following image:
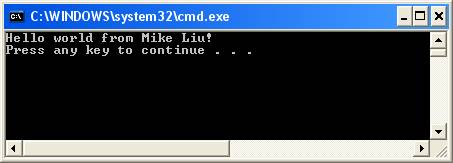
Setting the service application to AutoStart
Because we know we have to start the service before we
run the client program, we can make some changes to the solution to automate
this task; that is, to automatically start the service immediately before we run
the client program.
To do this, in the Solution Explorer, right-click on the
Solution, select Properties from the context menu, and you will
see the Solution 'HelloWorld' Property Pages dialog box.
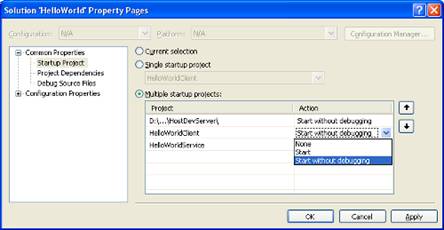
On this page, first select the option button Multiple
startup projects. Then, change the action of D:...HostDevServer to
Start without debugging. Change the HelloWorldClient to the same
action.
The HostDevServer must be above HelloWorldClient. If it
is not, use the arrows to move it to the top.
To try it, first stop the service, and then press
Ctrl+F5. You will notice that the HostDevServer is started first, and then the
client program runs without errors.
Note that this will only work inside Visual Studio IDE.
If you start the client program from Windows Explorer
(D:SOAwithWCFandLINQProjectsHelloWorld
HelloWorldClientbinDebugHelloWorldClient.exe) without first starting the
service, the service won't get started automatically and you will get an error
message saying 'Could not connect to http://localhost:8080/HostDevServer/
HelloWorldService.svc'.
Summary
In this article, we have implemented a basic WCF
service, hosted it within the ASP.NET Development Server, and created a command
line program to reference and consume this basic WCF service. At this point, you
should have a thorough understanding as to what a WCF is under the hood. You
will benefit from this when you develop WCF services using Visual Studio WCF
templates, or automation guidance packages. The key points covered in this
article are:
Ø
A WCF service is a class library, which defines one or more WCF
service interface contracts
Ø
System.ServiceModel assembly is referenced by all of the
WCF service projects
Ø
The implementations of a WCF service are just regular C#
classes
Ø
A WCF service must be hosted in a hosting application
|