Using RadioButtonList
control
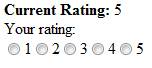
Using DropDownList control
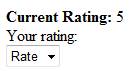
Read the below FAQ’s to have a quick understanding on jQuery library.
What is jQuery?
How to use it in ASP.Net Pages?
How to enable
jQuery intellisense in Visual Studio 2008?
How to use
jQuery intellisense in an external javascript file?
and the below article,
Introduction to
jQuery in ASP.Net and 10 Advantages to Choose jQuery
I will create the rating control as
user control so that it can be used anywhere in your project.
Steps
1. Open Visual Studio 2008.
2. Create a new “ASP.Net Web Site”.
3. Include a new user control in your project by right clicking the
solution and clicking “Add New Item...” dialog box. Select Web user control and
rename it accordingly. I have named it as SimpleRating.ascx.
4. Grab the new jquery library from here. Create a folder called
_scripts and copy the jquery library. Refer the above FAQ’s to know
more.
Building Rating Control
Since, we use jquery library and Ajax
method in our rating control we will need the item id in the client side to
record the rating against the item in the server. For this purpose, we will
include a HiddenField in our user control which can hold the itemid to the
client side. We will also include a panel control to populate the rating
control (DropDownList or RadioButtonList) depending on the preference set in the
rating control.
Refer the below code,
SimpleRating.ascx
<%@ Control Language="C#"
AutoEventWireup="true" CodeFile="SimpleRating.ascx.cs" Inherits="SimpleRating"
%>
<div class="Rating">
<asp:HiddenField ID="hfItemID"
runat="server" />
<div
id="dvCurrentRate"><b>Current Rating:</b> <% =CurrentRating
%></div>
Your rating:
<asp:Panel ID="plRateCtrl"
runat="server">
</asp:Panel>
</div>
In our user control, we will expose the
below 5 public properties to configure the rating control.
1. MaxRate
This takes
an integer and will dictate the maximum rate the rating control will
allow.
2. ItemID
This
property will hold the ID of the item against which you want to rate. For
example, if it is news site it will be newsid, article id for article site,
etc.
3. CurrentRating
Set this
property with the current average rating for the item when rendering the page to
the user. See the above figure.
4. IsReadOnly
This will
make the rating control to readonly.
For
example, set this property to true if the user has already rated this item. Set
it to false if you want the user to rate only after authenticating.
5. RatingControl
This takes
either DropDownList or RadioButton and renders the rating control accordingly in
the Panel.
Refer the below code,
SimpleRating.ascx.cs
using System;
using
System.Collections.Generic;
using System.Web;
using System.Web.UI;
using
System.Web.UI.WebControls;
public partial class SimpleRating :
System.Web.UI.UserControl
{
int _maxrate = 5;
RateControl _rateControl =
RateControl.DropDownList;
bool _isReadOnly = false;
int _currentRate;
int _itemid;
public int MaxRate
{
get
{
return _maxrate;
}
set
{
_maxrate = value;
}
}
public int ItemID
{
get
{
return _itemid;
}
set
{
_itemid = value;
hfItemID.Value =
value.ToString();
}
}
public int CurrentRating
{
get
{
return
_currentRate;
}
set
{
_currentRate =
value;
}
}
public bool IsReadOnly
{
get
{
return _isReadOnly;
}
set
{
_isReadOnly =
value;
}
}
public RateControl
RatingControl
{
set
{
if
(!Page.IsPostBack)
{
_rateControl =
value;
if (value ==
RateControl.DropDownList)
{
DropDownList ddl = new
DropDownList();
ddl.Items.Add(new
ListItem("Rate", ""));
for (int i = 1; i
<= MaxRate; i++)
{
ddl.Items.Add(new
ListItem(i.ToString(), i.ToString()));
}
if
(IsReadOnly)
ddl.Enabled =
false;
plRateCtrl.Controls.Add(ddl);
}
else
{
RadioButtonList
rdbList = new RadioButtonList();
rdbList.RepeatLayout =
RepeatLayout.Flow;
rdbList.RepeatDirection = RepeatDirection.Horizontal;
for (int i = 1; i
<= MaxRate; i++)
{
rdbList.Items.Add(new ListItem(i.ToString(), i.ToString()));
}
if
(IsReadOnly)
rdbList.Enabled =
false;
plRateCtrl.Controls.Add(rdbList);
}
}
}
}
protected void Page_Load(object
sender, EventArgs e)
{
if (!IsReadOnly)
{
if
(!Page.ClientScript.IsClientScriptIncludeRegistered("jquery"))
Page.ClientScript.RegisterClientScriptInclude("jquery",
"_scripts/jquery-1.4.1.min.js");
if
(!Page.ClientScript.IsClientScriptIncludeRegistered("RatingEngine"))
Page.ClientScript.RegisterClientScriptInclude("RatingEngine",
"_scripts/SimpleRating.js");
}
else
{
plRateCtrl.Enabled =
false;
}
}
}
public enum RateControl
{
DropDownList,
RadioButton
}
In the above code, setting DropDownList
in RatingControl property will render dropdownlist control in the panel and
setting RadioButtonList will render RadioButtonList control in the panel. We
will use jquery to read the user rating and sending it to server.
To record the rating, we will create a
simple HttpHandler which will accept itemid and user rating to record into the
database. Refer the below code,
RateHandler.ashx
<%@ WebHandler Language="C#"
Class="RateHandler" %>
using System;
using System.Web;
using System.Text;
public class RateHandler : IHttpHandler
{
public void ProcessRequest
(HttpContext context) {
//If user is not authenticated
then return, dont save rating!
//if
(!context.Request.IsAuthenticated)
//{
// return;
//}
string ItemID =
context.Request.QueryString["ItemID"];
string Rate =
context.Request.QueryString["Rate"];
string user =
context.User.Identity.Name;
//Insert the rating to database
and return the average of ratings for the itemid
//In your SP, check if the user
has already rated to prevent duplicate rating
int CurrentRating = 3; /*Should be
returned from SP*/
//Return the average rating in JSON
to client
StringBuilder jsonOutput = new
StringBuilder();
jsonOutput.AppendLine("[{");
jsonOutput.AppendLine("\"Rate\" :
\"" + CurrentRating.ToString() + "\"}]");
context.Response.Write(jsonOutput);
}
public bool IsReusable {
get {
return false;
}
}
}
|