This will give a better user experience when the
registration form is bigger and also will reduce the user’s effort to retype
certain form fields like password, etc again and again because password fields
will be reset in every submit.
Moving forward, we will see how to develop this feature
in ASP.Net using Ajax
methods in jQuery library.
Steps
1. Open Visual Studio 2008, Create a new ASP.Net Website.
2. Select a language of your
choice in Language dropdownlist. Name your website as per your need.
3. Download the latest jQuery
library from jQuery.com and integrate it into your project. The following
Faq’s will help you to that.
What is
jQuery? How to use it in ASP.Net Pages?
How to
enable jQuery intellisense in Visual Studio 2008?
How to use
jQuery intellisense in an external javascript file?
4. Include a new SQL express
database inside App_Data folder and create a table called tblLogin with
necessary columns.
Note
You can add database using the “Add New Item” dialog. Then, create a table
called tblLogin with the necessary columns using the “Server Explorer”.
Refer the below figure,
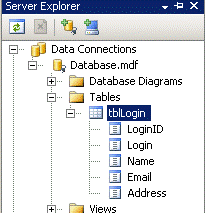
5. Next, we will create an
HttpHandler which will accept the desired username in querystring and check the
tblLogin table for the availability. To create an HttpHandler, right click the
project in your solution explorer and click “Add New Item”. Select “Generic
Handler”. Rename it to LoginHandler.ashx and click OK. Refer the below code,
<%@ WebHandler Language="C#" Class="LoginHandler"
%>
using System;
usingSystem.Web;
usingSystem.Data.SqlClient;
usingSystem.Configuration;
public class LoginHandler : IHttpHandler {
public void ProcessRequest (HttpContext context) {
string uname = context.Request["uname"];
string result = "0";
if (uname != null)
{
result = CheckUNAvailable(uname);
}
context.Response.Write(result);
context.Response.End();
}
public string CheckUNAvailable(string name)
{
string result = "0";
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["Sql"].ConnectionString);
try
{
con.Open();
SqlCommand com = new SqlCommand("Select Login from tblLogin
where Login=@Login", con);
com.Parameters.AddWithValue("@Login", name);
objectuname = com.ExecuteScalar();
if (uname != null)
result = "1";
}
catch
{
result = "error";
}
finally
{
con.Close();
}
return result;
}
publicboolIsReusable {
get {
return false;
}
}
}
Web.Config
<connectionStrings>
<add name="Sql" connectionString="Data
Source=.\SQLEXPRESS;AttachDbFilename=|DataDirectory|\Database.mdf;Integrated
Security=True;User Instance=True;"/>
</connectionStrings>
6. Now, we will consume this
HttpHandler using jquery in Default.aspx and will provide appropriate message to
let users know about the username availability.
|