To understand better, we will develop a small picture
album application where we will create a JavaScript array of image urls in code
behind file and will emit it to the HTML output.
How to declare JavaScript array from code behind?
The ClientScriptManager class has packed with a method
called RegisterArrayDeclaration() which can be used to register a JavaScript
array from code behind file. The ClientScript property of Page object will
expose the instance of ClientScriptManager object. The below code will register
a JavaScript array of image urls.
Picture Album using Javascript Arrays
protected void Page_Load(object sender, EventArgs e)
{
String arrName = "AlbumPicArray";
String arrValue =
"'/jsArrDemo/cartoons/1.bmp', '/jsArrDemo/cartoons/2.bmp','/jsArrDemo/cartoons/3.bmp', '/jsArrDemo/cartoons/4.bmp', '/jsArrDemo/cartoons/5.bmp'";
ClientScriptManager cs = Page.ClientScript;
cs.RegisterArrayDeclaration(arrName, arrValue);
}
When executed, the RegisterArrayDeclaration() will check
whether a registered array exists with the same name as specified in the
arrayName parameter and, if so, it will add the values specified in the
arrayValue parameter else, it will create a new JavaScript array with the name
and value specified. Once executed, you can see the JavaScript array declared in
the html output. Refer below,
<script type="text/javascript">
//<![CDATA[
var AlbumPicArray = new
Array('/cartoons/1.bmp','/cartoons/2.bmp','/cartoons/3.bmp','/cartoons/4.bmp','/cartoons/5.bmp');
//]]>
</script>
Now, you can go ahead and use the above JavaScript array
and can do your client side manipulations. In this article, I will use jQuery
library to implement the picture album using JavaScript array. If you are new to
jQuery library then I recommend you to take a quick look on the below FAQ’s to
understand this article better.
What is
jQuery? How to use it in ASP.Net Pages?
How to
enable jQuery intellisense in Visual Studio 2008?
How to
use jQuery intellisense in an external javascript file?
In this sample, we will provide a feature where user can
click Next and Previous link to navigate picture album. Something like
below,
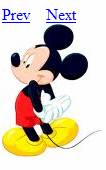
The below jQuery code will help you to achieve the
same.
|