The Numeric Converter allows you to convert a number of
any bases to another base. The method DecimalToBase, it converts a number from
Decimal to the specified base entered by the user. Another method BaseToDecimal
converts the number from the specified base to the corresponding decimal
equivalent.
The following method is not actually modified from the
previous version and is left as it is to convert a number to any base user has
entered.
string DecimalToBase(int iDec, int
numbase)
{
string strBin = "";
int[] result = new
int[32];
int MaxBit = 32;
for (; iDec > 0; iDec /=
numbase)
{
int rem = iDec %
numbase;
result[--MaxBit] =
rem;
}
for (int i = 0; i <
result.Length; i++)
if
((int)result.GetValue(i) >= base10)
strBin +=
cHexa[(int)result.GetValue(i) % base10];
else
strBin +=
result.GetValue(i);
strBin = strBin.TrimStart(new
char[] { '0' });
return strBin;
}
The method BaseToDecimal is modified for there was an
un-necessary check for hexa-decimal input.
int BaseToDecimal(string sBase, int
numbase)
{
int dec = 0;
int b;
int iProduct = 1;
string sHexa = "";
if (sBase.IndexOfAny(cHexa)
>= 0)
for (int i = 0; i <
cHexa.Length; i++)
sHexa +=
cHexa.GetValue(i).ToString();
for (int i = sBase.Length - 1;
i >= 0; i--, iProduct *= numbase)
{
string sValue =
sBase[i].ToString();
if
(sValue.IndexOfAny(cHexa) >= 0)
b =
iHexaNumeric[sHexa.IndexOf(sBase[i])];
else
b = (int)sBase[i] -
asciiDiff;
dec += (b *
iProduct);
}
return dec;
}
Both the methods are activated according to the user
inputs and invoked by pressing the Left Soft key for “Convert”.
if (comboBox1.SelectedItem.ToString() !=
"10" && comboBox2.SelectedItem.ToString() != "10")
{
String temp =
BaseToDecimal(textBox1.Text, Int32.Parse(comboBox1.Text)).ToString();
textBox3.Text =
DecimalToBase(Int32.Parse(temp), Int32.Parse(comboBox2.Text));
}
else if (comboBox2.SelectedItem.ToString()
== "10")
textBox3.Text =
BaseToDecimal(textBox1.Text, Int32.Parse(comboBox1.Text)).ToString();
else if (comboBox1.SelectedItem.ToString()
== "10")
textBox3.Text =
DecimalToBase(Int32.Parse(textBox1.Text),
Int32.Parse(comboBox2.Text));
Some sample outputs:
Converting a decimal to Binary
number:
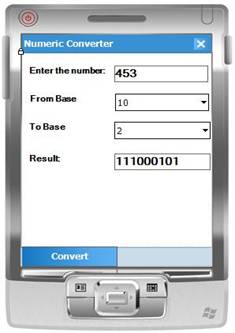
Converting a decimal to octal
number:
|