When a user selects a row, we will show
the details of the selected row in a DetailsView control by passing the selected
row’s ID to server and binding it. Something like below,
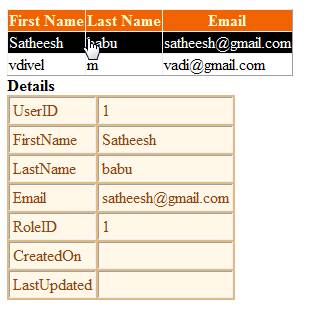
We will use __doPostBack() method to
post the page to server and bind the DetailsView control. Read my article Raising
Postback using __doPostBack() from Javascript in Asp.Net to know more
about __doPostBack() method. Obviously, we will use jQuery for the client side
interactions.
If you are new to jQuery, i advise you
to read the below FAQ’s to have a quick understanding.
What
is jQuery? How to use it in ASP.Net Pages?
How
to enable jQuery intellisense in Visual Studio 2008?
How
to use jQuery intellisense in an external javascript file?
Read the below article, to know more
about jQuery library and its advantages,
Introduction
to jQuery in ASP.Net and 10 Advantages to Choose jQuery
Moving forward, we will build the
master details view with the selectable GridView, DetailsView and
jQuery.
Steps
1. Open Visual Studio 2008.
2. Click New> Website and Select “ASP.Net Web Site”. You can select
the language of your choice. I have selected C#. Rename the website name as per
your need.
3. Include a new SQL express database inside App_Data folder and create
a table called Users with necessary columns.
Note
You can add
database by right clicking App_Data folder in the solution and clicking Add New
Item. This will bring a dialog box where you need to select “Sql Server
Database” and click Add. Just right click the added database and click “Open” to
open your database in Server Explorer on the left pane of your Visual Studio
2008. Then, create a table called Users with the necessary columns using the
“Server Explorer”.
4. Drag a GridView control from data tab of visual studio and name it as
gvUsers. Drag a DetailsView control to populate the details of a selected
row.
5. Include new DataBound columns to display the columns in the GridView
control. I have included 3 columns for First name, Last name and Email. Set the
primary key field to DataKeyNames property of the GridView control to identify
the row user has selected. Refer below,
<asp:GridView ID="gvUsers"
runat="server" AutoGenerateColumns="False"
DataKeyNames="UserID">
<Columns>
<asp:BoundField
DataField="FirstName" HeaderText="First Name" ReadOnly="True" />
<asp:BoundField
DataField="LastName" HeaderText="Last Name" ReadOnly="True" />
<asp:BoundField DataField="Email" HeaderText="Email" ReadOnly="True"
/>
</Columns>
<FooterStyle
BackColor="White" ForeColor="#330099" />
<RowStyle
BackColor="White" ForeColor="#330099" />
<SelectedRowStyle
BackColor="#FFCC66" Font-Bold="True" ForeColor="#663399" />
<PagerStyle
BackColor="#FFFFCC" ForeColor="#330099" HorizontalAlign="Center"
/>
<HeaderStyle
BackColor="#F06300" Font-Bold="True" ForeColor="#FFFFCC" />
</asp:GridView>
<b>Details</b>
<asp:DetailsView ID="DetailsView1"
runat="server" Height="50px" Width="125px"
BackColor="#DEBA84"
BorderColor="#DEBA84" BorderStyle="None" BorderWidth="1px"
CellPadding="3"
CellSpacing="2">
<FooterStyle
BackColor="#F7DFB5" ForeColor="#8C4510" />
<RowStyle BackColor="#FFF7E7"
ForeColor="#8C4510" />
<PagerStyle ForeColor="#8C4510"
HorizontalAlign="Center" />
<HeaderStyle
BackColor="#A55129" Font-Bold="True" ForeColor="White" />
<EditRowStyle
BackColor="#738A9C" Font-Bold="True" ForeColor="White" />
</asp:DetailsView>
6. Now, bind the GridView control from the codebehind by fetching the
data from users table.
protected void Page_Load(object sender,
EventArgs e)
{
if (!IsPostBack)
BindUsers();
}
public void BindUsers()
{
DataTable dt =
GetUsersForModeration();
gvUsers.DataSource =
dt;
gvUsers.DataBind();
}
public DataTable
GetUsersForModeration()
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["Sql"].ConnectionString);
con.Open();
SqlCommand com = new
SqlCommand("SP_GetUsersForModeration", con);
com.CommandType =
CommandType.StoredProcedure;
SqlDataAdapter ada = new
SqlDataAdapter(com);
DataSet ds = new
DataSet();
ada.Fill(ds);
return ds.Tables[0];
}
Execute the page. You can see the Users
data displayed in the GridView.
7. Since, we are not displaying the primary key field to the users in
GridView control we need to send the key value associated with every row to the
client so that we can identify the clicked row from client side. To do this, we
will add id property to every row of GridView control except the header and
assign the primary key field value as value to the id property.
protected void gvUsers_RowDataBound(object
sender, GridViewRowEventArgs e)
{
if (e.Row.RowType ==
DataControlRowType.DataRow)
{
string key =
gvUsers.DataKeys[e.Row.RowIndex].Value.ToString();
e.Row.Attributes.Add("id",
key);
}
}
Now, the rendered HTML table will
include id property to every TR tag. Refer below,
<table cellspacing="0" rules="all"
border="1" id="gvUsers" style="border-collapse:collapse;">
<tr
style="color:#FFFFCC;background-color:#F06300;font-weight:bold;">
<th
scope="col">First Name</th><th scope="col">Last
Name</th><th scope="col">Email</th>
</tr><tr
id="1" style="color:#330099;background-color:White;">
<td>Satheesh</td><td>babu</td><td>satheesh@gmail.com</td>
</tr><tr
id="2" style="color:#330099;background-color:White;">
<td>vadivel</td><td>m</td><td>vadi@gmail.com</td>
</tr><tr
id="3" style="color:#330099;background-color:White;">
<td>John</td><td>m</td><td>John@gmail.com</td>
</tr><tr
id="4" style="color:#330099;background-color:White;">
<td>Peter</td><td>m</td><td>Peter@gmail.com</td>
</tr><tr
id="5" style="color:#330099;background-color:White;">
<td>Kavi</td><td>m</td><td>Kavi@gmail.com</td>
</tr><tr
id="6" style="color:#330099;background-color:White;">
<td>Freddy</td><td>m</td><td>Freddy@gmail.com</td>
</tr><tr
id="7" style="color:#330099;background-color:White;">
<td>martin</td><td>m</td><td>martin@gmail.com</td>
</tr>
</table>
8. Next, we will integrate the jquery library and make the GridView
row(TR tag) selectable. Refer the FAQ [What
is jQuery? How to use it in ASP.Net Pages?] above to integrate the jQuery
library.
9. To make every row selectable, we can add a click event to every TR
except header. Refer the below code,
<style type="text/css">
.SelectedRow
{
background-color:Black;
color: White;
}
.Row
{
background-color: White;
color: Black;
}
</style>
<script
src="_scripts/jquery-1.4.1.min.js"
type="text/javascript"></script>
<script
type="text/javascript">
$(function() {
$('#<%=gvUsers.ClientID%>
tr[id]').mouseover(function() {
$(this).css({ cursor: "hand",
cursor: "pointer" });
});
$('#<%=gvUsers.ClientID%>
tr[id]').click(function() {
$('#<%=gvUsers.ClientID%>
tr[id]').attr("class","Row");
$(this).attr("class",
"SelectedRow");
});
});
</script>
|