Now in the AdminDAL of TESTDAL add this code
using System;
using
System.Collections.Generic;
using System.Text;
using
System.Data.SqlClient;
using System.Data;
using
System.Collections;
namespace TEST.DAL
{
public class AdminDAL
{
static SqlConnection conn;
static bool connected;
private static void
Connect()
{
int count =
0;
try
{
conn = new
SqlConnection("User ID=sa; Initial
Catalog=Northwind;Integrated Security=SSPI;Persist Security Info=False; Data
Source=localhost");
try
{
if
(!connected)
{
conn.Open();
}
}
catch
(Exception ex)
{
}
}
catch (SqlException ex)
{
}
return;
}
private static void
closeconnection()
{
if
(connected)
conn.Close();
}
public static ICollection
GetData()
{
DataSet ds =
new DataSet();
if
(!connected)
Connect();
SqlDataAdapter
da = new SqlDataAdapter("", conn);
string query =
"SELECT Title,FirstName,LastName from Employees";
da.SelectCommand = new SqlCommand(query, conn);
da.Fill(ds, "Categories");
return
ds.Tables["Categories"].Rows;
}
}
For this code to work you need to include the following
namespace.
using
System.Data.SqlClient;
using System.Data;
using
System.Collections;
Since, we have used ICollection in our code the
namespace System.Collections should be added. To make the namespace more user
friendly name we can rename it to TEST.DAL.
The above code will connect to the database using
Connect() method. We can call closeconnection() method to close the connection.
The method GetData() is used to fetch data from Northwind database table
“Employees”.
Now build the TESTDAL project as shown below
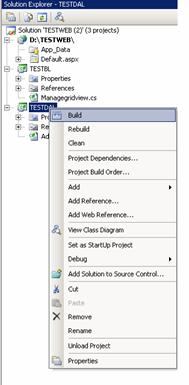
Once done, we need to add the DAL project to BL project.
Right click on the TESTBL project and choose “AddReferance”.
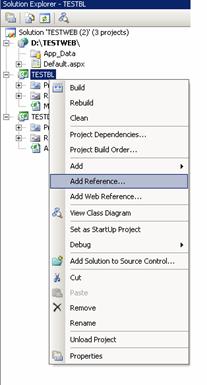
Reach Projects tab and choose TESTDAL project as shown
in the below figure.
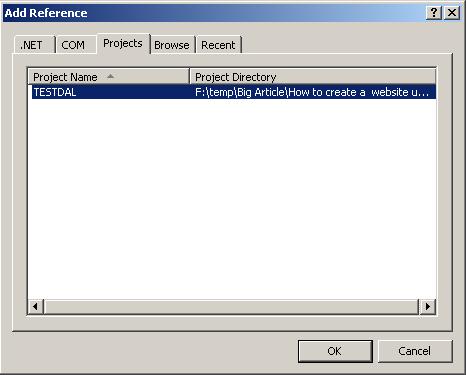
Next, we will build the business logic layer.
Open the class file Managegridview.cs and add the
following code,
using System;
using
System.Collections.Generic;
using
System.Collections;
using System.Text;
using TEST.DAL;
namespace TEST.BL
{
public class Managegridview
{
public static ICollection
GetData()
{
return AdminDAL.GetData();
}
}
}
We have to include the following namespaces
using
System.Collections;
using TEST.DAL;
In the above code, we have used ICollection so we have
include the namespace System.Collections. Inorder to access the functions in
AdminDAL we have to include Test.DAL namespace as well.
When we open the managegridview class file for the first
time, you will get TESTBL which has to be replaced with TEST.BL for the same
reason I have pointed fotd for DAL.
Build the project TESTBL. This project now needs to be
accessed from website to bind the gridview and hence we need to add it to our
website. To do this, Right click the website project in solution explorer choose
“Add Reference” and reach Projects tab and choose TESTBL project as shown in the
below figure.
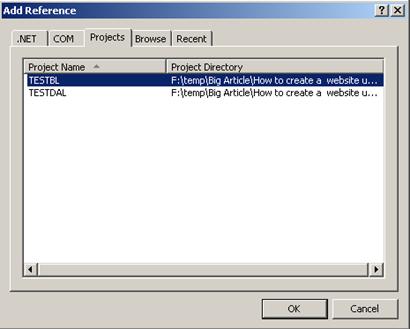
In the Default.apx page, add a gridview control. Refer
the below aspx code,
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" runat="server" Width="600px" HeaderStyle-BackColor="blue" RowStyle-BackColor="AliceBlue" CellPadding="4" ForeColor="#333333" GridLines="None">
<FooterStyle BackColor="#990000" Font-Bold="True" ForeColor="White" />
<RowStyle BackColor="#FFFBD6" ForeColor="#333333" />
<SelectedRowStyle BackColor="#FFCC66" Font-Bold="True" ForeColor="Navy" />
<PagerStyle BackColor="#FFCC66" ForeColor="#333333" HorizontalAlign="Center" />
<HeaderStyle BackColor="#990000" Font-Bold="True" ForeColor="White" />
<AlternatingRowStyle BackColor="White" />
</asp:GridView>
</div>
</form>
In the code behind, we need to call the Business logic
layer’s GetData() method to bind the GridView. Refer the below code,
using System;
using System.Data;
using
System.Configuration;
using System.Web;
using
System.Web.Security;
using System.Web.UI;
using
System.Web.UI.WebControls;
using
System.Web.UI.WebControls.WebParts;
using
System.Web.UI.HtmlControls;
using
System.Collections;
using TEST.BL;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if
(!Page.IsPostBack)
{
ICollection
colcat = Managegridview.GetData();
if
(colcat.Count > 0)
{
GridView1.DataSource = ((DataRowCollection)colcat)[0].Table;
GridView1.DataBind();
}
}
}
In the above code, we are getting the data by calling
GetData() method of BL layer which inturns fetches the data from database by
calling DAL layer.
when you run the website,you will have output as shown
below
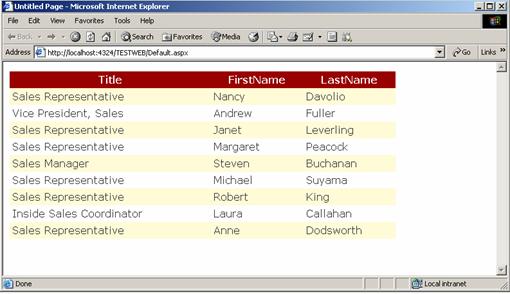
|