Moving forward, we will understand on
how to use this control and some of its advanced usages.
Before moving to the actual
implementation we will try to understand the XML file format which the AdRotator
control can understand to render the ads.
XML file format
As said earlier, the XML file will
contain the advertisement information. It has an outer element called
<Advertisements> which packs number of advertisements under <Ad>
tag. Refer below,
<?xml version="1.0" encoding="utf-8"
?>
<Advertisements>
<Ad>
// ad 1
</Ad>
<Ad>
// ad 2
</Ad>
<Ad>
// ad 3
</Ad>
</Advertisements>
In the above format, each <Ad>
element will contain information about each ad. Below is list of element we can
define for each element.
ImageUrl
The absolute or relative URL to an
image file.
NavigateUrl
The destination URL of a page to link
to if the user clicks the ad.
Height
The height of the image, in
pixels.
Width
The width of the image, in
pixels.
AlternateText
The ALT text display in place of the
image when the image specified by the ImageUrl property is not
available.
Keyword
A category or a keyword describing the
advertisement.
Impressions
A number that indicates the importance
of the ad in the schedule of rotation relative to the other ads in the file.
Higher the number, then higher the number of times the ad will be rendered by
the control.
Note
1. The total of all <Impressions> values in the XML file cannot
exceed 2,047,999,999. If it does, the AdRotator control throws a run-time
exception.
2. Apart from the above elements, we can also define some custom tags(We
will see more about this in later section of this article.
3. All the above elements are optional. Hence, you can choose the
required one for your ad.
With this information, we will move
forward and build a simple page that displays ads with AdRotator
control.
Steps
1. Create a new Asp.Net website.
2. Add a XML file to your solution through “Add new item..” dialog box.
To secure the xml file from downloading you can add it to App_Data folder. Any
file inside this folder will throw “HTTP Error 403 – Forbidden” error when tried
accessing.
3. Now, add some advertisements using above format. Refer
below,
<?xml version="1.0" encoding="utf-8"
?>
<Advertisements>
<Ad>
<ImageUrl>~/img/AccordionPanel.jpg</ImageUrl>
<NavigateUrl>
http://www.codedigest.com/Articles/jQuery/335_Creating_Accordion_Panel_ using_jQuery_in_ASPNet_%e2%80%93_Part_1.aspx
</NavigateUrl>
<AlternateText>
Creating Accordion Panel using
jQuery in ASP.Net
</AlternateText>
</Ad>
<Ad>
<ImageUrl>~/img/Menu.gif</ImageUrl>
<NavigateUrl>
http://www.codedigest.com/Articles/ASPNET/340_ASPNET_Horizontal_Menu_Control.aspx
</NavigateUrl>
<AlternateText>
Creating ASP.NET Horizontal Menu
Control
</AlternateText>
</Ad>
</Advertisements>
4. Drag an AdRotator control from visual studio toolbox.
5. Configure AdvertisementFile property of AdRotator control to the XML
file. Refer below,
<asp:AdRotator id="AdRotator1"
runat="server"
Target="_new"
AdvertisementFile="~/App_Data/Ads/AdsXML.xml"/>
6. Press F5 to execute the project.
Displaying Advertisements from
Database in AdRotator control
Maintaining the advertisements in XML
file is not a great idea when you want to change or update the ads very often.
It will be really good if we can manage the ads from database. AdRotator
control has the capability to achieve this through a normal databinding or by
using DataSource controls. To do this, we need to create a table with the XML
tag names as column names. Refer below,
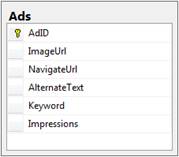
You can then use a DataSource control
and bind the AdRotator control through its DataSourceID property. The below code
does that,
<asp:AdRotator ID="AdRotator1"
runat="server" DataSourceID="SqlDataSource1" />
<asp:SqlDataSource
ID="SqlDataSource1" runat="server"
ConnectionString="<%$
ConnectionStrings:ConnectionString %>"
SelectCommand="SELECT * FROM
[Ads]"></asp:SqlDataSource>
At times, we might want to display the
ad with some filter condition or to put it in other words, to display some
relevant ads on a page. For example, if you have a page that has some
information about ASP.Net then displaying ads that is specific to ASP.Net will
be a great thing. We will see about this in next section.
|