What is the advantage?
The readability of our code increases.
Gives compile time error for datatype mismatch.
With visual studio you will get the intellisense support for
tables and columns.
Going ahead we will see how to create a simple typed dataset
and using it with the help of visual studio. I am using NorthWind database to
explain typed dataset in this article.
Creating typed dataset:
1) Open a web application project in visual studio 2005.
2) To add a typed dataset right click the solution and click
“Add New Item”. It will open new item dialog box like below (Figure 1),
Figure 1 – Add New Item
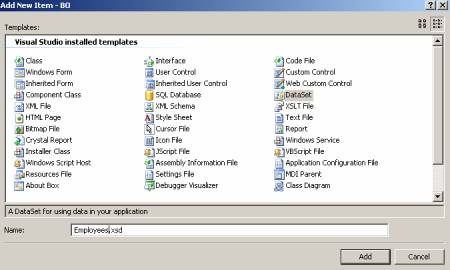
3) Select Dataset and change the name, click Add.
4) Open server explorer in visual studio, if you can’t find
the server explorer click “Server Explorer" from the view menu of visual studio.
5) It will open Add Connection window. Select the Server name
and select the “NorthWind” database or your own database as below,
Figure 2 – Add Connection
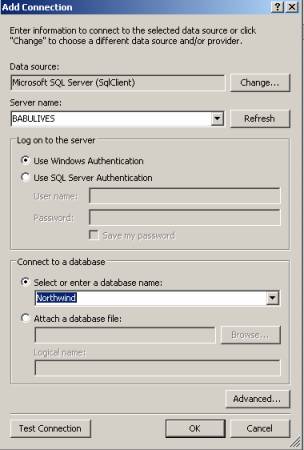
Select Server name and select the database name and click
OK.
6) Drag and drop Employees table into the dataset designer to
create the typed dataset from the server explorer. The designer will look like,
Figure 3 – Dataset Designer
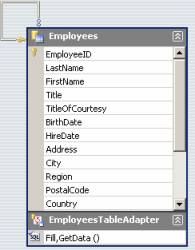
By default it will have a TableAdapter (EmployeesTableAdapter
here) with Fill() and GetData() methods that can be used to fill and fetch data
from the database without implementing a line of code.
Intellisense Support in visual Studio:
Visual studio helps us with the intellisense support (Refer
Figure 4) by listing tables, columns and methods for the constructed typed
dataset.
Figure 4 – Visual Studio Intellisense
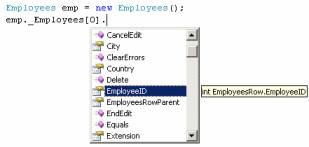
“_Employees[0]” in the intellisense is the table name and “_”
is because we used same name for the dataset name as the table name. If the
Dataset name is different then the table name will be “Employees”.
How to use it?
We can use the typed dataset and fill it in normal way as we
do for a non typed dataset.
Listing 2: Filling Typed Dataset in a usual way
com = new SqlCommand("Select * from Employees", sqlcon);
ada = new SqlDataAdapter(com);
emp = new Employees();
da.Fill(emp,"Employees");
The table name in fill method should match the table name in
typed dataset.
Using EmployeesTableAdapter,
Listing 3: Filling Typed Dataset using TableAdapter
BO.EmployeesTableAdapters.EmployeesTableAdapter ada = new
BO.EmployeesTableAdapters.EmployeesTableAdapter();
gvEmployees.DataSource = ada.GetData();
gvEmployees.DataBind();
In the above code “BO” is the namespace name since I have
used separate Class library project with name BO and created the typed dataset
there. If you are creating typed dataset in the same web project then the above
code will be,
Listing 4: Filling Typed Dataset using TableAdapter in Same
project
EmployeesTableAdapters.EmployeesTableAdapter ada = new
EmployeesTableAdapters.EmployeesTableAdapter();
gvEmployees.DataSource = ada.GetData();
gvEmployees.DataBind();
here “gvEmployees” is the gridview. In the next coming
sections we will see how to use TableAdapter and extend the typed dataset to
provide custom methods that serves for specific purpose. We use “customer” table
in NorthWind database for this article.
Figure 5 – Customer Table
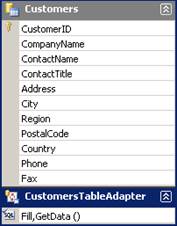
What is a TableAdapter?
TableAdapters provide communication between your application
and a database. More specifically, a TableAdapter connects to a database,
executes queries or stored procedures, and either returns a new data table
populated with the returned data or fills an existing DataTable with the
returned data. TableAdapters are also used to send updated data from your
application back to the database.
Adding New Functionality:
We will try to add a new Selection functionality and a new
update functionality using TableAdapter in Typed dataset using visual
studio.
New Select Functionality:
To retrieve a particular customer details by giving the
CustomerID.
Right click dataset click add>Query as shown in below
figure.
Figure 6 – Customer Table
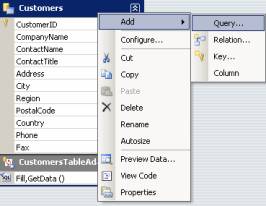
This will bring a window like in the Figure 7,
Figure 7 – TableAdapter Configuration for Command Type
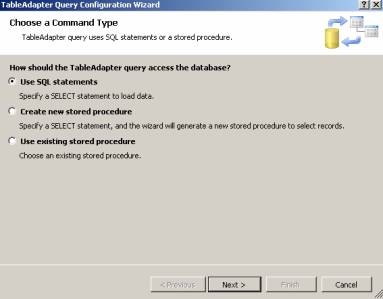
Select appropriate option, I have selected “Use SQL
statements”. Click Next.
Clicking Next will bring a window like,
Figure 8 – TableAdapter Configuration for Query Type
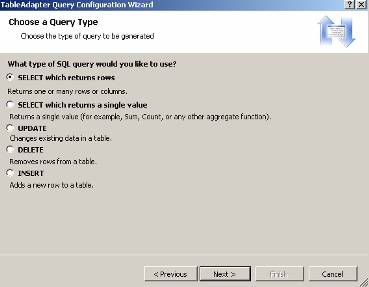
Select the query type. I have selected the first option
“Select which returns rows”. Click Next.
This will bring a window to specify the query. Give the
parameters with “@” character pre-pended to it.
Figure 9 – TableAdapter Configuration for SQL Statement
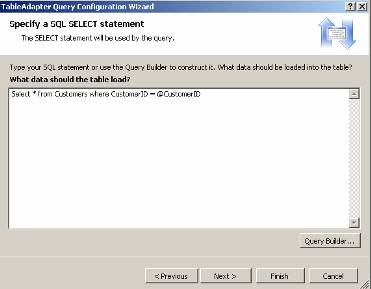
I would like to return details of a customer on giving the
customerid in this example so I have given the query like,
Select * from Customers where CustomerID = @CustomerID
Click Finish. Thus, we have added a select functionality to
the typed dataset using TableAdapter writing a single line of code.
New Update Functionality:
Repeat the above steps to update the address of the
customers. Select “Update” option in step 3, provide a method name in next step
and give the following query that will update address, city, region, postalcode,
country, phone and fax by giving the customerid.
The update query will be,
UPDATE [Customers] SET [Address] = @Address, [City] =
@City, [Region] = @Region, [PostalCode] = @PostalCode, [Country] = @Country,
[Phone] = @Phone, [Fax] = @Fax WHERE (([CustomerID] = @Original_CustomerID))
So our final dataset in designer will look like,
Figure 10 – Final Customer table view
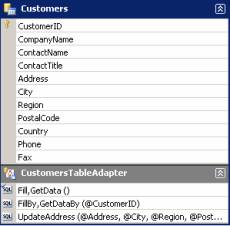
How to use the functionality?
To execute the above functionality instantiate Table adapter
like,
Listing 5: Instantiating TableAdapter
TestTableAdapters.CustomersTableAdapter ada = new
TestTableAdapters.CustomersTableAdapter();
Now we can see the new select and update functionality with
the insert, update and delete method in TableAdapter intellisense.
Figure 11 – Visual studio intellisense for TableAdapter
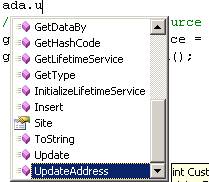
We can use the above functionality like,
Listing 6: Using the new functionality
//Update Address
ada.UpdateAddress("2nd Cross", "Bangalore", "Karnataka",
"560061", "India", "123456789", "!23456789", "100");
//Get details by customerid
grdCustomers.DataSource = ada.GetDataBy("100");
grdCustomers.DataBind();
In the next coming section we will construct a custom typed
dataset using visual studio instead of dragging and dropping from server
explorer.
|