In a real business world, it will not
be appropriate to use these default predefined templates and functionalities to
achieve our goal. This means, we will require our own customizations done on the
Dynamic Data Website for incorporating our business logic to customizing the UI
for business branding needs, etc. The good thing about Dynamic Data Website is,
it has a greater flexibility to customize it to a very granular level. We can
incorporate our business rules wherever required, can change the display of
particular database object, fields, etc.
Moving forward, we will see how to
build a custom page for a particular database object while the other database
objects can still use the default templates. Apart from providing the CRUD
operations, we will further customize the page to provide simple search
functionality on the database rows.
To understand better, we will use the
same sample application which we discussed in my previous article.
Steps
1. Open Visual Studio 2008.
2. Click New> Website and Select “Dynamic Data Web Site”. The project will have some aspx and ascx files created
automatically by default. Refer the below figure.
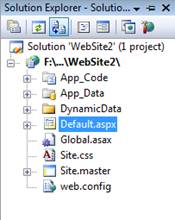
3. Include a new SQL express database inside App_Data folder and create
a table called Employees and Department using the visual studio's Server Explorer.
Refer the below figure.
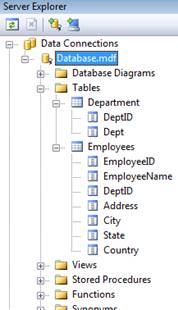
4. Design your LINQ to SQL classes. Read
Introduction to LINQ to SQL – A Beginners Guide if you are new to LINQ to SQL.
Click Save on the LINQ to SQL designer window after creating LINQ to SQL objects.
Refer the below figure.
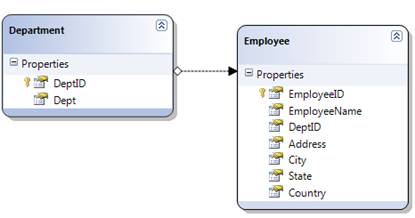
6. Next, open the Global.asax file. You will find a method called RegisterRoutes()
in Application_Start event. Refer the below code that can be found in
Global.asax file for the method.
public static void
RegisterRoutes(RouteCollection routes) {
MetaModel model = new
MetaModel();
// IMPORTANT:
DATA MODEL REGISTRATION
// Uncomment this line to register
LINQ to SQL classes or an ADO.NET Entity Data
// model for ASP.NET Dynamic Data.
Set ScaffoldAllTables = true only if you are sure
// that you want all tables in the
data model to support a scaffold (i.e. templates)
// view. To control scaffolding
for individual tables, create a partial class for
// the table and apply the
[Scaffold(true)] attribute to the partial class.
// Note: Make sure that you change
"YourDataContextType" to the name of the data context
// class in your
application.
//model.RegisterContext(typeof(YourDataContextType), new ContextConfiguration()
{ ScaffoldAllTables = false });
// The following statement
supports separate-page mode, where the List, Detail, Insert, and
// Update tasks are performed by
using separate pages. To enable this mode, uncomment the following
// route definition, and comment
out the route definitions in the combined-page mode section that
follows.
routes.Add(new
DynamicDataRoute("{table}/{action}.aspx") {
Constraints = new
RouteValueDictionary(new { action = "List|Details|Edit|Insert" }),
Model = model
});
// The following statements
support combined-page mode, where the List, Detail, Insert, and
// Update tasks are performed by
using the same page. To enable this mode, uncomment the
// following routes and comment
out the route definition in the separate-page mode section above.
//routes.Add(new
DynamicDataRoute("{table}/ListDetails.aspx") {
// Action =
PageAction.List,
// ViewName =
"ListDetails",
// Model = model
//});
//routes.Add(new
DynamicDataRoute("{table}/ListDetails.aspx") {
// Action =
PageAction.Details,
// ViewName =
"ListDetails",
// Model = model
//});
}
Update the “YourDataContextType” in the above auto generated code with your data context class(
EmployeeDataClasses in my case) as said in the code comments.
Also, make the property
ScaffoldAllTables to true. The final code will be,
model.RegisterContext(typeof(EmployeeDataClassesDataContext), new
ContextConfiguration() { ScaffoldAllTables = true });
Save the file and execute the application to see the
basic CRUD operations on the datamodel in action.
Next, we will create custom page with
search functionality only for Employee object.
To do this, we need to create a folder
inside the DynamicData\CustomPages with the name of the object (by adding “s” at
the end) for which we need to create the custom page. In our case, for our
employee object create a folder called “Employees”. Now, to make our custom page
creation easy, we can copy the list.aspx from “PageTemplates” folder to our
Employees folder. We can now start customizing our page. Refer the below figure
to understand better.
|