6.
Find the RegisterRoutes() method in Application_Start event of your Global.asax file. You will find the below code
for RegisterRoutes() method in Global.asax file.
public static void
RegisterRoutes(RouteCollection routes) {
MetaModel model = new
MetaModel();
// IMPORTANT:
DATA MODEL REGISTRATION
// Uncomment this line to register
LINQ to SQL classes or an ADO.NET Entity Data
// model for ASP.NET Dynamic Data.
Set ScaffoldAllTables = true only if you are sure
// that you want all tables in the
data model to support a scaffold (i.e. templates)
// view. To control scaffolding
for individual tables, create a partial class for
// the table and apply the
[Scaffold(true)] attribute to the partial class.
// Note: Make sure that you change
"YourDataContextType" to the name of the data context
// class in your
application.
//model.RegisterContext(typeof(YourDataContextType), new ContextConfiguration()
{ ScaffoldAllTables = false });
// The following statement
supports separate-page mode, where the List, Detail, Insert, and
// Update tasks are performed by
using separate pages. To enable this mode, uncomment the following
// route definition, and comment
out the route definitions in the combined-page mode section that
follows.
routes.Add(new
DynamicDataRoute("{table}/{action}.aspx") {
Constraints = new
RouteValueDictionary(new { action = "List|Details|Edit|Insert" }),
Model = model
});
// The following statements
support combined-page mode, where the List, Detail, Insert, and
// Update tasks are performed by
using the same page. To enable this mode, uncomment the
// following routes and comment
out the route definition in the separate-page mode section above.
//routes.Add(new
DynamicDataRoute("{table}/ListDetails.aspx") {
// Action =
PageAction.List,
// ViewName =
"ListDetails",
// Model = model
//});
//routes.Add(new
DynamicDataRoute("{table}/ListDetails.aspx") {
// Action =
PageAction.Details,
// ViewName =
"ListDetails",
// Model = model
//});
}
Just uncomment the first line of code (bolded) and configure your data context class name in the place of
“YourDataContextType” on the above code, EmployeeDataClasses in my case.
Also, make the property
ScaffoldAllTables to true. The final code will be,
model.RegisterContext(typeof(EmployeeDataClassesDataContext), new
ContextConfiguration() { ScaffoldAllTables = true });
This completes the creation of fully operational CRUD website on our data model.
Execute the application and see it in action.
Next, we will go ahead and customize
the Boolean template in the default field templates folder. Browse to
DynamicData/FieldTemplates and open Boolean.ascx. Now, comment the CheckBox
control in the ascx and add a Literal control. You can see the code where the
database value is actually rendered in the CheckBox control in the codebehind of
the usercontrol through FieldValue property. Now, assign value as “Yes” if the
FieldValue property returns true else “No” to the Literal control. Refer the
below code,
Boolean.ascx
<%@ Control Language="C#"
CodeFile="Boolean.ascx.cs" Inherits="BooleanField" %>
<%--<asp:CheckBox runat="server"
ID="CheckBox1" Enabled="false" />--%>
<asp:Literal ID="Literal1"
runat="server"></asp:Literal>
Boolean.ascx.cs
public partial class BooleanField :
System.Web.DynamicData.FieldTemplateUserControl {
protected override void
OnDataBinding(EventArgs e) {
base.OnDataBinding(e);
object val =
FieldValue;
if (val != null)
{
// CheckBox1.Checked = (bool)
val;
Literal1.Text = ((bool)val ?
"Yes" : "No");
}
}
public override Control DataControl
{
get {
// return CheckBox1;
return Literal1;
}
}
}
That’s it! Execute the application and
you can see Boolean field shows “Yes” or “No” in view mode and CheckBox control
in edit and insert mode. Refer the below figure,
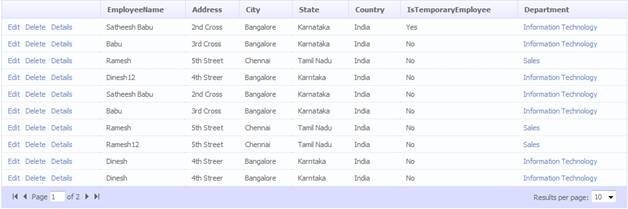
In the above figure,
IsTemporaryEmployee field is Boolean which uses the customized field template.
Note
Department field uses ForeignKey.ascx
template and View Employees in Department object uses Children.ascx as i said
earlier.

Because we have not customized the edit
and insert field template, edit page will still show CheckBox control. Refer
below figure,
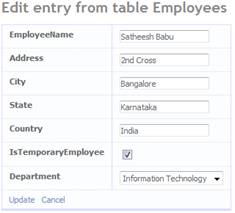
|