Moving forward, we will see how this
editor can be used in ASP.Net projects.
Steps
1. From your Start menu, open Visual Studio 2008, Click File >Website and choose ASP.Net
Website with the language of your choice and create a new website project.
2. Download the latest TinyMCE files from here. The current version
is 3.3b2.
3. Unzip the zip file. Copy the TinyMCE folder into your visual studio
solution. Refer below,
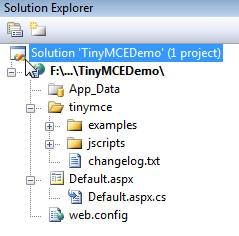
4. Drag an ASP.Net textbox control into your aspx page. Set its TextMode
property to MultiLine.
5. To use the TinyMCE features with the MultiLine textbox, we need to
include Tiny_MCE.js file which is under the folder tinymce/jscripts/tiny_mce/ of
the package into our webpage.
Refer
below,
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
Make sure you give the
correct path of the above javascript file from your solution.
6. Next, we need to call the TinyMCE javascript API to make the
multiline text box to an editor instance. Refer the code below,
<head runat="server">
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
<script type="text/javascript"
language="javascript">
tinyMCE.init({
mode: "textareas"
});
</script>
<title></title>
</head>
7. That’s it! Execute the page and you can see the TinyMCE in action. By
default, the TinyMCE editor will include a simple toolbar set with some basic
toolset.
Refer
below,
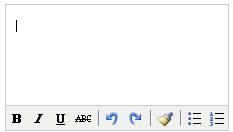
The final code will look
like,
<head runat="server">
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
<script type="text/javascript"
language="javascript">
tinyMCE.init({
mode: "textareas"
});
</script>
<title></title>
</head>
<body>
<form id="form1"
runat="server">
<div>
<asp:TextBox ID="TextBox1"
TextMode="MultiLine" runat="server"></asp:TextBox>
</div>
</form>
</body>
</html>
The default editor instance (Refer the
image above) we get will have a theme called “Simple”. Hence, the above is code
is equivalent to,
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
<script type="text/javascript"
language="javascript">
tinyMCE.init({
mode: "textareas",
theme: "simple"
});
</script>
Note
Please note that the above code will
make all the textarea in the page to rich text editor. We will see how to
restrict this behaviour in coming sections.
Adding Advanced Toolbar
Options
To make the TinyMCE editor to have all
the advanced styling options, the editor includes one another theme called
Advanced which can be applied.
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
<script type="text/javascript"
language="javascript">
tinyMCE.init({
mode: "textareas",
theme: "advanced"
});
</script>
When executed, we will have an editor
similar to below,
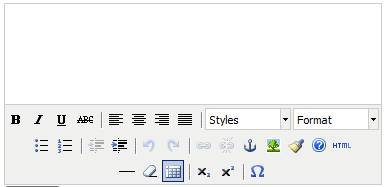
With this knowledge, we will move
forward and do more customization on the TinyMCE editor.
Customizing TinyMCE editor
The TinyMCE editor includes some HTML
examples in root/tinymce/examples/ folder which we can refer to customize the
editor. In the TinyMCE package in solution, browse to
root/tinymce/examples/full.html. Now, copy the tinyMCE.init() function which
includes all the options to our page, Default.aspx.
Refer the code below,
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
<script type="text/javascript"
language="javascript">
tinyMCE.init({
// General options
mode: "textareas",
theme: "advanced",
plugins:
"safari,pagebreak,style,layer,table,save,advhr,advimage,advlink,
emotions,iespell,inlinepopups,insertdatetime,preview,media,
searchreplace,print,contextmenu,
paste,directionality,fullscreen,noneditable,visualchars,nonbreaking,
xhtmlxtras,template,wordcount",
// Theme options
theme_advanced_buttons1:
"save,newdocument,|,bold,italic,underline,strikethrough,|,
justifyleft,justifycenter,justifyright,justifyfull,styleselect
,formatselect,fontselect,fontsizeselect",
theme_advanced_buttons2:
"cut,copy,paste,pastetext,pasteword,|,search,replace,|,bullist,numlist,|
,outdent,indent,blockquote,|,undo,redo,|,link,unlink,
anchor,image,cleanup,help,code,|,insertdate,inserttime,
preview,|,forecolor,backcolor",
theme_advanced_buttons3:
"tablecontrols,|,hr,removeformat,visualaid,|,sub,sup,|,charmap,emotions,
iespell,media,advhr,|,print,|,ltr,rtl,|,fullscreen",
theme_advanced_buttons4:
"insertlayer,moveforward,movebackward,absolute,|,
styleprops,|,cite,abbr,acronym,del,ins,attribs,|,visualchars,nonbreaking,
template,pagebreak",
theme_advanced_toolbar_location:
"top",
theme_advanced_toolbar_align:
"left",
theme_advanced_statusbar_location:
"bottom",
theme_advanced_resizing:
true,
// Example content CSS (should be
your site CSS)
content_css:
"css/content.css",
// Drop lists for
link/image/media/template dialogs
template_external_list_url:
"lists/template_list.js",
external_link_list_url:
"lists/link_list.js",
external_image_list_url:
"lists/image_list.js",
media_external_list_url:
"lists/media_list.js",
// Replace values for the template
plugin
template_replace_values:
{
username: "Some
User",
staffid: "991234"
}
});
</script>
|
|
Execute the page and see the editor
with all the available options in action.
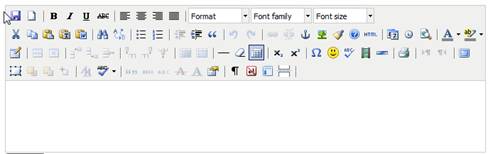
You can now start customizing the
editor from the above code easily.
In the above code, you can see the
properties that can be set for controlling the toolbar location and alignment,
status bar location, resizable option of the editor. Refer below,
theme_advanced_toolbar_location:
"top",
theme_advanced_toolbar_align:
"left",
theme_advanced_statusbar_location:
"bottom",
theme_advanced_resizing:
true,
You can start removing the unwanted
toolbars styles, plugins to just satisfy your requirements. Use the property
theme_advanced_buttonsN to define your toolbar set, where N is line number the
toolbar needs to be displayed.
For example, to customize a editor to
look like below,
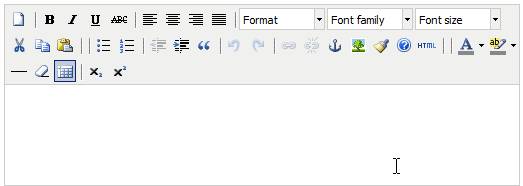
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
<script type="text/javascript"
language="javascript">
tinyMCE.init({
// General options
mode: "textareas",
theme: "advanced",
// Theme options
theme_advanced_buttons1:
"save,newdocument,|,bold,italic,underline,strikethrough,
|,justifyleft,justifycenter,
justifyright,justifyfull,|,formatselect,fontselect,fontsizeselect",
theme_advanced_buttons2:
"cut,copy,paste,pastetext,pasteword,|,search,replace,
|,bullist,numlist,|,outdent,indent,
blockquote,|,undo,redo,|,link,unlink,anchor,image,cleanup,help,code,
|,insertdate,inserttime,preview,|,forecolor,backcolor",
theme_advanced_buttons3:
"hr,removeformat,visualaid,|,sub,sup",
theme_advanced_toolbar_location:
"top",
theme_advanced_toolbar_align:
"left"
});
</script>
Disable TinyMCE editor
The codes discussed in the above
sections will convert all the textareas in the page to TinyMCE rich text editor.
Often, we will require making only some selected textareas in the page as rich
text editor. To do this, TinyMCE editor has a property called editor_deselector
which can be initialized with a class name. You can now restrict a textarea by
applying the initialized class name with the class property of the
textarea.
Refer the below code,
<head runat="server">
<script type="text/javascript"
src="tinymce/jscripts/tiny_mce/tiny_mce.js"></script>
<script type="text/javascript"
language="javascript">
tinyMCE.init({
// General options
mode: "textareas",
theme: "advanced",
editor_deselector:
"NoEditor",
// Theme options
theme_advanced_buttons1:
"save,newdocument,|,bold,italic,underline,strikethrough,|
,justifyleft,justifycenter,justifyright,
justifyfull,|,formatselect,fontselect,fontsizeselect",
theme_advanced_buttons2:
"cut,copy,paste,pastetext,pasteword,|,search,replace,
|,bullist,numlist,|,outdent,indent,blockquote,
|,undo,redo,|,link,unlink,anchor,image,cleanup,help,code,
|,insertdate,inserttime,preview,|,forecolor,backcolor",
theme_advanced_buttons3:
"hr,removeformat,visualaid,|,sub,sup",
theme_advanced_toolbar_location:
"top",
theme_advanced_toolbar_align:
"left"
});
</script>
<title></title>
</head>
<body>
<form id="form1"
runat="server">
<div>
<B>Rich Text
Editor</B>
<asp:TextBox ID="TextBox1"
TextMode="MultiLine" runat="server"></asp:TextBox>
<B>Regular
TextArea</B>
<asp:TextBox ID="TextBox2"
TextMode="MultiLine" CssClass="NoEditor"
runat="server"></asp:TextBox>
</div>
When executed you can see the output
like below,
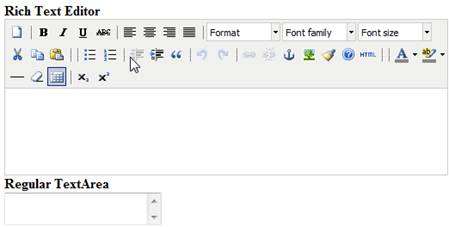
Fetching the Text from TinyMCE
Editor
Since, it is an ASP.Net textbox control
it can be accessed by regular Text property in the server. By default, ASP.Net
does not allow HTML inputs to be posted to server in order to prevent the cross
site scripting attach. You will get the following error,
A potentially dangerous Request.Form value
was detected from the client (TextBox1="").
You need to set ValidateRequest="false"
in the Page directive to prevent this error.
|