Accessing <html> elements in code
There are set of new classes in .Netframework 2.0 that are added in the
System.Web.UI.HtmlControls Namespace which make this
feasible. Moving forward, we will comprehend some of the HTML features that can
be accessed in codebehind through this new classes.
Accessing <Head> tag
It can be done through HtmlHead class that is packed in
the namespace System.Web.UI.HtmlControls. The Page object gives us a way through
Header property to access the <head> element.
For example,
HtmlHead head = Page.Header;
Setting Page Title
With the help of HtmlHead class, it is possible to set
the page title from the codebehind.
Below code will help us understanding it.
HtmlHead head = Page.Header;
HtmlTitle title = new HtmlTitle();
title.Text = "Test Page";
head.Controls.Add(title);
In short, the above requirement can be achieved by,
HtmlHead head = Page.Header;
head.Title = "Test Page";
This code will add a <title> tag in <head>
element.
Constructing CSS Styles from CodeBehind
We can add stylesheet elements in <head> tag of the HTML output generated by asp.net from the
codebehind class, and these constructed styles can be applied to the controls on the
page. Next section will help us achieving it.
Generic styles
We can create generic css styles and apply it to all the
controls without applying the style explicitly to the controls. For example,
creating a css class for input tag and applying to all the input tags in the
page can be done by,
HtmlHead head = Page.Header;
Style inputStyle = new Style();
inputStyle.ForeColor = System.Drawing.Color.Red;
inputStyle.BackColor = System.Drawing.Color.LightGray;
head.StyleSheet.CreateStyleRule(inputStyle, null,
"input");
The above code will generate a HTML output like,
<html xmlns="http://www.w3.org/1999/xhtml" >
<head><title>
Test Page
</title><style type="text/css">
input { color:Red;background-color:LightGrey; }
</style></head>
<body>
<form name="form1" method="post" action="Default.aspx"
id="form1">
<div>
<input name="TextBox1" type="text" id="TextBox1"
/></div>
</form>
</body>
</html>
So, all the textbox in the page will be applied with the
above style.
The output will be like,
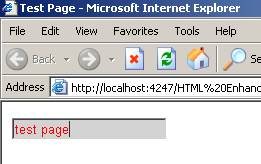
Applying Styles explicitly to a control
We can create css styles specific for controls and apply
it to the controls explicitly. The ASP.Net controls now have ApplyStyle(Style)
and MergeStyle(Style) methods packed with the control to achieve the same.
Style textStyle = new Style();
textStyle.ForeColor = System.Drawing.Color.DarkRed;
textStyle.BorderColor = System.Drawing.Color.DarkBlue;
textStyle.BorderWidth = 2;
Page.Header.StyleSheet.RegisterStyle(textStyle, null);
TextBox1.MergeStyle(textStyle);
The above code will generate an html output similar to
below,
<html xmlns="http://www.w3.org/1999/xhtml" >
<head><title>
Untitled Page
</title><style type="text/css">
.aspnet_s0 {
color:DarkRed;border-color:DarkBlue;border-width:2px;border-style:solid; }
</style></head>
<body>
<form name="form1" method="post" action="Default2.aspx"
id="form1">
<div>
<input name="TextBox1" type="text" id="TextBox1"
class="aspnet_s0" />
</div>
</form>
</body>
</html>
Output will be,
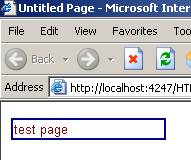
Adding <Link> tag
The link tag is useful to register a CSS file/Jscript
with our Webpage. It can be achieved by HtmlLink class.
Refer the below code that adds a stylesheet file from
codebehind.
HtmlLink cssLink = new HtmlLink();
cssLink.Href = "StyleSheet.css";
cssLink.Attributes.Add("rel", "stylesheet");
cssLink.Attributes.Add("type", "text/css");
Page.Header.Controls.Add(cssLink);
This will inject a <link> in the html output
inside the <head> tag.
<link href="StyleSheet.css" rel="stylesheet"
type="text/css" />
Adding <Meta> tag
Meta tags can be used to construct search engine
friendly websites, can be used to refresh page and many more. In ASP.Net 2.0, we
can achieve this from codebehind and hence we have the capability to add in
runtime.
The following code will do it,
HtmlHead head = (HtmlHead)Page.Header;
HtmlMeta metasearch1 = new HtmlMeta();
HtmlMeta metasearch2 = new HtmlMeta();
metasearch1.Name = "descriptions";
metasearch1.Content = "my personal site";
head.Controls.Add(metasearch1);
metasearch2.Name = "keywords";
metasearch2.Content = "ASP.Net,C#,SQL";
head.Controls.Add(metasearch2);
//Meta to refresh the page
HtmlMeta metarefresh = new HtmlMeta();
metarefresh.HttpEquiv = "refresh";
metarefresh.Content = "5";
head.Controls.Add(metarefresh);
The above code injects the following tags inside the
<head> element in the output html.
<meta name="descriptions" content="my personal site"
/>
<meta name="keywords" content="ASP.Net, C#, SQL"
/>
<meta http-equiv="refresh" content="5" />
Adding clientside Reset button
Sometimes, we will have requirements to reset the input
controls on the webpage from clientside. HtmlInputReset class will help us doing
it from codebehind class. The following code will add a reset input element in
the output html.
HtmlInputReset reset = new HtmlInputReset();
reset.ID = "ResetButton";
reset.Value = "Reset";
PlaceHolder1.Controls.Add(reset);
The above code will add a reset tag like below,
<input name="ResetButton" type="reset" id="ResetButton"
value="Reset" />
This class is new in 2.0 while the same can be achieved
through HtmlInputButton class in 1.x.
|