Figure 1 – Tiled Layout using ListView
control
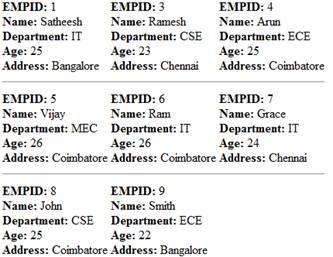
As i said in my previous article, we
need to define the LayoutTemplate and ItemTemplate at the minimum to display
data using ListView control. If we want to group data, then additionally we need
to define the GroupTemplate and specify the GroupItemCount property of ListView
control. We will understand this in coming sections.
GroupTemplate
The GroupTemplate will dictate how the
data can be grouped. For example, to group data like the above figure we should
define a TR tag with 3 TD’s. In this case, the grouping element will be TR.
Thus, GroupTemplate should have TR which in turn should populate the items in TD
using ItemTemplate.
GroupItemCount
This property will dictate the number of
items to repeat in each group. In our example, it is 3 TD’s.
We can understand this when we build a
sample ListView control with grouping. Next section will help you to do
that.
Steps
1. You can create a new Asp.Net website project
with C# as language in your Visual Studio 2008.
2. Include a new SQL express database inside App_Data folder and create
a table called Users with necessary columns.
Refer the below figure,
Figure 2 – Server Explorer
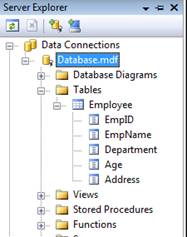
3. Drag a ListView control and SqlDataSource control from the data tab
of visual studio.
4. Configure the SqlDataSource control to fetch the Employee table data
from the Sql Express database under App_Data folder.
<asp:SqlDataSource ID="SqlDataSource1"
runat="server"
ConnectionString="<%$
ConnectionStrings:ConnectionString %>"
SelectCommand="SELECT * FROM
[Employee]"></asp:SqlDataSource>
Web.config
<connectionStrings>
<add
name="ConnectionString" connectionString="Data
Source=.\SQLEXPRESS;AttachDbFilename=|DataDirectory|\Database.mdf;Integrated
Security=True;User Instance=True"
providerName="System.Data.SqlClient"/>
</connectionStrings>
5. As is said earlier, to display a tiled layout(Grouping) we need to
design the GroupTemplate additionally to LayoutTemplate and ItemTemplate. Refer
the below code,
<asp:ListView ID="lvEmp"
runat="server"
DataSourceID="SqlDataSource1"
GroupItemCount="3">
<LayoutTemplate>
<table>
<tr
ID="groupPlaceholder" runat="server">
</tr>
</table>
</LayoutTemplate>
<GroupTemplate>
<tr>
<td
ID="itemPlaceholder" runat="server">
</td>
</tr>
</GroupTemplate>
<ItemTemplate>
<td
align="left">
<b>EMPID:</b> <asp:Label ID="EmpIDLabel" runat="server"
Text='<%# Eval("EmpID") %>' /><br />
<b>Name:</b> <asp:Label ID="EmpNameLabel" runat="server"
Text='<%# Eval("EmpName") %>' /><br />
<b>Department:</b> <asp:Label ID="DepartmentLabel" runat="server"
Text='<%# Eval("Department") %>' /><br />
<b>Age:</b> <asp:Label ID="AgeLabel" runat="server" Text='<%#
Eval("Age") %>' /><br />
<b>Address:</b> <asp:Label ID="AddressLabel" runat="server"
Text='<%# Eval("Address") %>' />
</td>
</ItemTemplate>
</asp:ListView>
In the above code, the ItemTemplate
content will be repeated 3 times(GroupItemCount) inside the GroupTemplate
element “itemPlaceholder” and it will be then copied to the
LayoutTemplate’s groupPlaceholder element.
6. Execute the page and you can see the Listview grouping in
action.
7. Now, to get the line just after every row (group) we need define an
additional template called GroupSeparatorTemplate.
Refer
below,
<GroupSeparatorTemplate>
<tr
runat="server">
<td
colspan="3"><hr /></td>
</tr>
</GroupSeparatorTemplate>
|