In short, ListView control can be
thought as a hybrid between GridView and Repeater control. ListView control
gives us more control on the rendered HTML output with edit/update/delete
feature. Also, ListView control has a built in support for adding new row,
sorting, etc. Refer the below figures which shows some of sample formats that
can be achieved through ListView control for a better understanding.
Sample Format 1
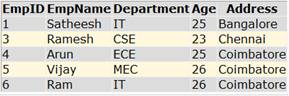
Sample Format 2
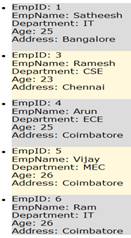
Sample Format 3

Features of ListView
Control
· Can
define our own template/Layout/Structure for the data display.
· Edit/Update/Delete capabilities on the data displayed.
· Built-in support for inserting new row.
· Built-in support for sorting
· Supports databinding via DataSource Controls including LINQ
DataSource controls.
· Paging via DataPager control, which can be part of ListView control
or can be kept outside the control. Means, DataPager can be kept at any part of
page as opposed to GridView where the built-in paging is packed with the control
itself.
Using ListView Control
In this
section, we will learn to use the ListView control with a simple databinding. We
will create an Employee table and populate the data in ListView.
One thing
to note here is, the ListView by default will not output any HTML output hence
binding it to any datasource simply will not output anything. If you execute
the below code,
lvEmployee.DataSource =
GetEmployee("Select * from Employee");
lvEmployee.DataBind();
It will give the following
error,
An item placeholder must be specified on
ListView 'lvEmployee'. Specify an item placeholder by setting a control's ID
property to "itemPlaceholder". The item placeholder control must also specify
runat="server".
The similar code with GridView will
output the employee details without any error.
Since ListView is designed to provide
more flexibility and control on the rendered HTML it never outputs any HTML by
default. We need to provide the basic required templates for the ListView to
output the data and it should have an item placeholder tag with the attribute
runat=”server”.
Consider, we need to output the
employee table in a tabular view, refer Sample Format 1 in the above
figures.
The basic required templates for a
ListView control to display the data are,
· Layout Template
This is the
template which decides the Layout of the data display and it will have the root
element. It should have an item placeholder tag with runat=”server” attribute.
The item placeholder can be anything with runat=”server” attribute like,
<asp:PlaceHolder> control, DIV tag, table, etc. We can specify the headers
for the displayed data here. The default value for item placeholder is
“itemplaceholder”. If we change this name explicitly then we need to reference
this name in ItemPlaceholderID property of ListView.
· Item Template
This
template will have content to render the actual data.
There are many other optional templates
available for ListView control which can be used on need basis. So, with the
above code and defining these 2 minimum required templates will output the data
as Sample Format 1.
The Layout Template will be,
<table ID="itemPlaceholderContainer" >
<tr>
<th>
EmpID
</th>
<th>
EmpName
</th>
<th>
Department
</th>
<th>
Age
</th>
<th>
Address
</th>
</tr>
<tr runat="server" ID="itemPlaceholder">
</tr>
</table>
While rendering the output the ListView
control will emit all the data in item template in the itemplaceholder tag in
the above mark-up.
Item Template will be,
<tr>
<td>
<asp:Label ID="EmpIDLabel" runat="server"
Text='<%# Eval("EmpID") %>' />
</td>
<td>
<asp:Label ID="EmpNameLabel" runat="server"
Text='<%# Eval("EmpName") %>'
/>
</td>
<td>
<asp:Label ID="DepartmentLabel" runat="server"
Text='<%# Eval("Department") %>'
/>
</td>
<td>
<asp:Label ID="AgeLabel" runat="server"
Text='<%# Eval("Age") %>' />
</td>
<td>
<asp:Label ID="AddressLabel" runat="server"
Text='<%# Eval("Address") %>' />
</td>
</tr>
|