Pre-Requisites
To understand this article, create a new ASP.Net site
with the language of your choice in your Visual studio 2010. I have used C# in
this article. Now, download the latest version of log4net from its official site.
The latest version is 1.2.11 at the time of this writing. You can download the
zip file with new key as advised in the site. Unzip the file and you can find
the dll in the folder log4net-1.2.11-bin-newkey\log4net-1.2.11\bin\net
for each framework version. We will use the one in 4.0 folder for this
article. Add reference to the dll in your solution explorer. Please note that
you can use Nuget package manager also to get the log4net integrated in your
application.
Before moving to actual implementation it will be better
to know some of the basics of log4net framework to better understanding.
log4net framework
The log4net framework has 3 main components: logger,
appender and layout. A logger is the one which actually logs the error to a log
destination with the help of an appender. There are different types of appenders
available that writes to different sources. For example, Event log, a database
table, a text file, emails, etc. The layout component will define the structure
or format of the actual logs message that is logged.
The loggers can be assigned with various levels which
dictate if it should log or not to log a message. The levels have a priority as
specified below,
1. ALL
2. DEBUG
3. INFO
4. WARN
5. ERROR
6. FATAL
7. OFF
All these levels except ALL and OFF have an equivalent
logging method defined in the logger to log the message for each level.
1. void Debug(object message);
2. void Info(object message);
3. void Warn(object message);
4. void Error(object message);
5. void Fatal(object message);
When you set a logger to a priority level “ALL” then it
will log all the messages you specified in your code regardless the levels i.e.
it will log all the message in debug, info, warn, error, fatal methods. A logger
with priority level set to “Error” will log only the messages in Error and Fatal
logger methods. Similarly, if the logger level is set to OFF then no messages
with be logged. The below simple table will help you understand what gets logged
for each priority level.
Logger Method |
ALL |
DEBUG
|
INFO
|
WARN
|
ERROR
|
FATAL
|
OFF |
Debug(object message) |
x |
x |
|
|
|
|
|
Info(object message) |
x |
x |
x |
|
|
|
|
Warn(object message) |
x |
x |
x |
x |
|
|
|
Error(object message) |
x |
x |
x |
x |
x |
|
|
Fatal(object message) |
x |
x |
x |
x |
x |
x |
|
Configuring Logging
To configure logging using log4net in your Asp.Net
application you first need to configure an Appender with a Layout format defined
and a Logger in Web.config.
As a first step, register the config section named
log4net in <configSections> of your web.config.
<configSections>
<section name="log4net" type="log4net.Config.Log4NetConfigurationSectionHandler,
log4net"/>
</configSections>
Assuming we need to log the exceptions to EventLog
destination the configuration will be,
<log4net>
<appender name="EventLogAppender"
type="log4net.Appender.EventLogAppender" >
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date [%thread] %-5level %logger [%property{NDC}] -
%message%newline" />
</layout>
</appender>
<root>
<level value="ALL"/>
<appender-ref ref="EventLogAppender"/>
</root>
</log4net>
The above section should be configured outside
<system.web> element. The above setting configures a default logger called
root logger which will capture any log message raised in the code. You can also
create logger explicitly by naming them apart from creating a default logger
which is demonstrated in next part of this article. The default logger is set
to a level “ALL” which means the logger will log all the messages raised in the
application.
Just for demonstration purpose I will create a divide by
zero exception and log it using log4net logger methods. See below,
public partial class _Default : System.Web.UI.Page
{
private static readonly
ILog log = LogManager.GetLogger(typeof(_Default));
protected void Page_Load(object sender, EventArgs e)
{
int n1 = 10;
int n2 = 0;
double result2 =
n1 / n2;
Response.Write(result2.ToString());
}
public void Page_Error(object sender, EventArgs e)
{
Exception
objErr = Server.GetLastError().GetBaseException();
log.Fatal(objErr.Message, objErr);
}
}
When executed, the above code will log the exception to
Event Log as seen in below figure.
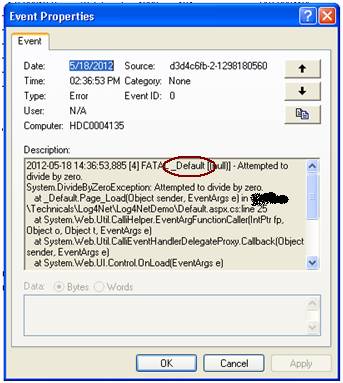
In the code, we can obtain the logger object using the
static method GetLogger(Type/Name) in LogManager class. Here the log4net creates
a logger with name _Default (codebehind class name) when we call
LogManager.GetLogger(typeof(_Default)) in the above code. The logger names are
usually appended in the log message which will helps us to identify the place
where the exception occurred easily. For example, _Default in the above figure
hints the exception occurred in _Default object. If the exception is occurred in
some other class then the name of the class specified as fully qualified name as
logger name will occur in the log message which hints us the exception location.
|