By default, whenever there are any
error occurred in the server side processing when using ASP.Net Ajax, the error
message is notified to the user using a message box (alert). The error message
that is displayed on the message box will be the actual error message contained
in the original exception’s Message property. In web applications, we
normally show an error page with a generic message to the user and log the
technical error information to a log file or database or email the error
message. This article will help us to customize the errors and notify the
users in a better way that gives a better user experience. Moving forward, we will see,
Ø Show a
Generic Error Message.
Ø Display Generic Error with Technical information.
Ø Displaying error message on the page instead of
MessageBox.
Ø Using
ASP.Net Error Handling Techniques.
Show a Generic Error Message
It will be better, if we suppress the
technical error message and instead show a generic error message to the user
when there is an error occurred in the server side processing. To do this,
ScriptManager object has a property called AsyncPostBackErrorMessage
which can be used to specify a generic error message.
For example,
ScriptManager1. AsyncPostBackErrorMessage
= “Error Occurred”;
This will always give “Error
Occurred!!” message for all the exceptions happened in server side processing.
Refer the below figure.
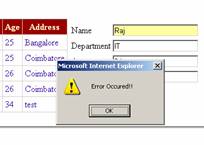
Display Generic Error with Technical
Information
There is an event called
AsyncPostBackError which will be called on the server when there is an
error occurred. We can set AsyncPostBackErrorMessage property of
ScriptManager object either declaratively or can be customized in this event.
e.Exception.Message property will give the actual technical error information
which can be logged or displayed to the user.
ASPX
<asp:ScriptManager ID="ScriptManager1"
runat="server" OnAsyncPostBackError="ScriptManager1_AsyncPostBackError"
/>
Codebehind
protected void
ScriptManager1_AsyncPostBackError(object sender, AsyncPostBackErrorEventArgs
e)
{
ScriptManager1.AsyncPostBackErrorMessage = "Error Occured!!\n" +
e.Exception.Message;
}
Refer the below figure for better
understanding.
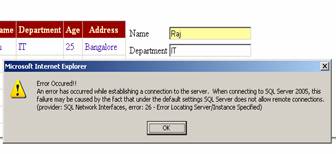
Displaying the error message on the page
instead of MessageBox
Every time when there is an error, it is
then notified to the users through a Message box in ASP.Net AJAX application. It will be better if
we suppress this message box and display the error information in the page
instead of the message box.
To do this, we can utilize the
endRequest() event of PageRequestManager object which manages the AJAX in the
client side. We can suppress the message box and display the actual error in the
page through this event. To get an instance of PageRequestManager, we can call
the method Sys.WebForms.PageRequestManager.getInstance().
To display the error message in the
page instead of a message box we can use the following script.
<script type="text/JavaScript"
language="JavaScript">
function pageLoad()
{
var manager =
Sys.WebForms.PageRequestManager.getInstance();
manager.add_endRequest(endRequest);
}
function endRequest(sender,
args)
{
var Error =
args.get_error();
document.getElementById("divError").innerHTML = Error.message;
args.set_errorHandled(true);
} </script>
<div id="divError" style="color:Red"
></div>
|