Making GridView Row selectable using JavaScript
When we want to add Delete, Select functionality to a
grid we normally proceed by adding a button/hyperlink for each action. By
capturing the ID of the row in each action i.e. in ItemCommand or RowCommand
(2.0) we can complete the action. To make users more comfortable we can make the
whole gridview row selectable so that we can get rid of Delete and Select
button/hyperlink from the grid using Jscript. Refer the below figure.
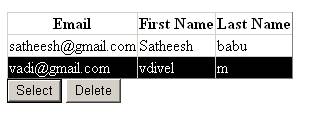
How to achieve this?
We can simulate the selection of a row by calling
Javascript function that makes the background of the clicked row to a different
color. Refer the above figure. Also, we will declare a hidden field to populate
the ID of selected gridview’s row from javascript. In Select/Delete button click
we can get the selected row’s ID from the hidden field and can complete the
required action.
<asp:GridView ID="gvUsers" runat="server"
AutoGenerateColumns="False" OnRowDataBound="gvUsers_RowDataBound">
<Columns>
<asp:BoundField DataField="Email" HeaderText="Email"
ReadOnly="True" />
<asp:BoundField DataField="FirstName" HeaderText="First
Name"
ReadOnly="True" />
<asp:BoundField DataField="LastName" HeaderText="Last
Name"
ReadOnly="True" />
</Columns>
</asp:GridView>
<!- - Hidden field -- >
<INPUT id="hdnEmailID" type="hidden" value="0"
runat="server" NAME="hdnEmailID">
Javascript functions:
<script language="javascript">
var lastRowSelected;
var originalColor;
function GridView_selectRow(row, EmailID)
{
var hdn=document.form1.hdnEmailID;
hdn.value = EmailID;
if (lastRowSelected != row)
{
if (lastRowSelected != null)
{
lastRowSelected.style.backgroundColor =
originalColor;
lastRowSelected.style.color = 'Black'
lastRowSelected.style.fontWeight = 'normal';
}
originalColor = row.style.backgroundColor
row.style.backgroundColor = 'BLACK'
row.style.color = 'White'
row.style.fontWeight = 'normal'
lastRowSelected = row;
}
}
function GridView_mouseHover(row)
{
row.style.cursor = 'hand';
}
</script>
We can link the javascript function call from Gridview’s
Row in RowDataBound Event.
protected void gvUsers_RowDataBound(object sender,
GridViewRowEventArgs e)
{
if (e.Row.RowType ==
DataControlRowType.DataRow)
{
e.Row.ID = e.Row.Cells[0].Text;
e.Row.Attributes.Add("onclick",
"GridView_selectRow(this,'" + e.Row.Cells[0].Text + "')");
e.Row.Attributes.Add("onmouseover",
"GridView_mouseHover(this)");
}
}
In Select/Delete button click event we can get the id of
the row clicked by,
hdnEmailID.Value
Thus, we can complete the required action with the id in
the hidden variable.
|