We will create the thumbnail image using HttpHandler
like we did in Part
1and Part
2.
Creating Thumbnail Image
Thumbnail.ashx
public void ProcessRequest (HttpContext context) {
string imageurl =
context.Request.QueryString["ImURL"];
string str = context.Server.MapPath(".") +
imageurl;
Bitmap bmp = new Bitmap(str);
System.Drawing.Image img = bmp.GetThumbnailImage(100, 100,
null, IntPtr.Zero);
MemoryStream ms = new MemoryStream();
img.Save(ms,
System.Drawing.Imaging.ImageFormat.Jpeg);
byte[] bmpBytes = ms.GetBuffer();
img.Dispose();
ms.Close();
context.Response.BinaryWrite(bmpBytes);
context.Response.End();
}
Note:
I have given only the ProcessRequest() method in the
above code. Refer the source code attachment for the full code.
In the above code, we are reading a BitMap image object
by reading the image from the file system. BitMap object has method called
GetThumbnailImage which accepts height and width to create thumbnail image from
the existing image. The created thumbnail image is then converted to byte
array(bmpBytes) using MemoryStream object and it is outputted by doing a binary
write.
We can do this through an aspx page, but I will use
HttpHandler to do this since we do not need some page level events. Read my
previous articles on HttpHandler linked in the reference section of this
article.
We can test run this HttpHandler by hard coding an
existing URL in the database.
Now, the created thumbnail image should be displayed in
a GridView column. When the user clicks this thumbnail image on the gridview, it
should open a popup window and display the full image. This can be done by
passing the image link as a querystring to an aspx page which has an Image
control. Next section will help us achieving the same.
Displaying the Original Image in a Popup Window
We will create page which accepts the image link as a
Querystring and assign it ImageUrl property of the image control.
Refer the code below,
FullImage.aspx
protected void Page_Load(object sender, EventArgs
e)
{
string imageurl = Request.QueryString["ImURL"];
string str = Server.MapPath(".") + imageurl;
Image1.ImageUrl = str;
}
Binding the GridView
We have the thumbnail HttpHandler and the full image
generating aspx page ready which needs to be binded with GridView. Refer the
below code,
GridView Code
<asp:GridView ID="gvImages" runat="server"
AutoGenerateColumns="False" CellPadding="4" ForeColor="#333333" GridLines="None"
>
<Columns>
<asp:BoundField HeaderText = "Image
Name" DataField="imagename" />
<asp:TemplateField
HeaderText="Image">
<ItemTemplate>
<a
href="javascript:void(window.open('FullImage.aspx?ImURL=<%#Eval("Image")%>','_blank','toolbar=no,menubar=no'))">
<asp:Image ID="Image1" runat="server" ImageUrl='<%#
"ThumbNailImage.ashx?ImURL="+Eval("Image") %>'/> </a>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<FooterStyle BackColor="#990000"
Font-Bold="True" ForeColor="White" />
<RowStyle BackColor="#FFFBD6"
ForeColor="#333333" />
<SelectedRowStyle BackColor="#FFCC66"
Font-Bold="True" ForeColor="Navy" />
<PagerStyle BackColor="#FFCC66"
ForeColor="#333333" HorizontalAlign="Center" />
<HeaderStyle BackColor="#990000"
Font-Bold="True" ForeColor="White" />
<AlternatingRowStyle BackColor="White"
/>
</asp:GridView>
When we execute the page, we will get an output similar
to below.
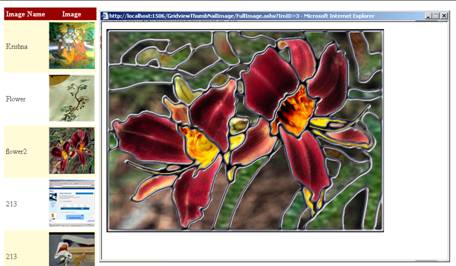
The main drawback of the above approach is, we are
opening the full image in a popup window and we all know that displaying
something on a popup window will not give a better user experience. There might
be a chance where the popup blocker is turned on in the user’s machine and will
not allow the user to view the full image. The next section will mitigate the
above drawback by displaying the full image in a DIV tag instead of a popup
window.
|