Sample Demonstrating the AutoCompleteExtender
Let’s say we have requirement where the user is
registering for an online and event and he needs to enter his current
designation, we need to display all the designations that are starting with
typed words on the textbox in a popup panel below
Steps
1. Drag a TextBox control and the
AutoCompleteExtender control on to the WebForm. Assign to the TargetControlID of
the AutoCompleteExtender to the ID of the Textbox. Also give the SeviceMethod
and ServicePath required to fetch the values. The markup looks like this:
<asp:TextBox ID="TextBox1" runat="server" ></asp:TextBox>
<ajaxToolkit:AutoCompleteExtender
ID="AutoCompleteExtender2"
BehaviorID="countries"
TargetControlID="TextBox1"
ServicePath="Designations.asmx"
ServiceMethod="GetDesignations"
MinimumPrefixLength="1"
runat="server">
</ajaxToolkit:AutoCompleteExtender>
2. Add a webservice to the
project, let’s say Designations.asmx and write the webmethod which fetches the
value from database.
Eg:
[WebMethod]
public string[] GetDesignations(string prefixText)
{
SqlConnection con = new
SqlConnection("server=local;integrated
security=true;database=master");
con.Open();
string strQuery =
"select * from designations where designation like
'" + prefixText + "%'";
DataSet ds = new DataSet();
SqlDataAdapter dta = new SqlDataAdapter(strQuery,con);
dta.Fill(ds);
con.Close();
List<string> desigsArList = new List<string>();
for (int i = 0; i < ds.Tables[0].Rows.Count; i++)
{
desigsArList.Add(ds.Tables[0].Rows[i]["designation"].ToString());
}
return
desigsArList.ToArray();
}
3. Assign the ScriptService
attribute to the class WebService class we are using:
Eg:
[System.Web.Script.Services.ScriptService]
public class Countries :
System.Web.Services.WebService
{
}
4. Now run the application and the
output looks as given in the below screenshot when typed a single character
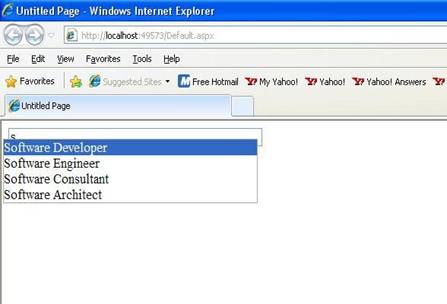
Displaying the popup without using the Webservice
We can also display completion list using
AutoCompleteExtender control and without using WebServices in the following
way:
1. First enable the PageMethods
for the scriptmanager. Check the sample markup below
<ajaxToolkit:ToolkitScriptManager runat="server" EnablePageMethods="true" ID="ToolkitScriptManager1" />
2. Now write the webmethod in the
codebehind file as shown below:
[WebMethod]
public static string[] GetCountriesList(string prefixText, int count)
{
List<string> countriesArList = new List<string>();
countriesArList.Add("India");
countriesArList.Add("Iran");
countriesArList.Add("Iraq");
countriesArList.Add("Israel");
return
countriesArList.ToArray();
}
//this is
a sample method which fetches static values beginning with “I”
Make sure the method is a static method and
follows all the rules required by AutoCompleteExtender.
3. Just assign the WebMethod to
the autocomplete extender as given below without using the service path.
<ajaxToolkit:AutoCompleteExtender
ID="AutoCompleteExtender1"
BehaviorID="AutoCompleteEx" TargetControlID="TextBox2"
ServiceMethod="GetCountriesList" MinimumPrefixLength="1"
runat="server"
FirstRowSelected="true" >
</ajaxToolkit:AutoCompleteExtender>
|