Data context can be set directly to a
common language runtime (CLR) object, with the bindings evaluating to properties
of that object. Alternatively, you can set the data context to a
DataSourceProvider object.
This dependency property inherits
property values. If there are child elements without other values for
DataContext established through local values or styles, then the property system
will set the value to be the DataContext value of the nearest parent element
with this value assigned.
Creating a Web Service
To demonstrate the purpose of this
article, we must first create an ASP.NET web service project named
“MyWebService” and add a custom class WebContent to the project.
This class has a string property, GreetingMessage and a field
Names, a string array.
public class WebContent
{
string greet;
public string
GreetingString
{
get
{
return greet;
}
set
{
greet = value;
}
}
public string[] Names;
}
For convenience, create a web service
method that sets the values of the properties and return the object of this
custom type.
Here is the code for the web service
definition:
public class Service1 :
System.Web.Services.WebService
{
[WebMethod]
public WebContent
CreateObject()
{
WebContent wc = new
WebContent();
wc.GreetingString = "Hello
World";
wc.Names = new string[5]
{"Bala", "Murali", "Balaji", "Krish", "Chris" };
return wc;
}
}
Build the web service project and do
the test run to execute the method.
Creating a WPF Window that consumes
the Web Service
Now to consume the web service created
above in WPF application, create a new WPF window project and add few text block
and listbox controls on to the form.
To call a web service, add a Service
Reference from the Project Menu and supply the URL (http://localhost/MyWebService/service1.asmx)
in the dialog shown.
Create a proxy for the web service
reference in the OnLoad event handler of the WPF window and call the
CreateObject method as below:
void OnLoad(object sender,
RoutedEventArgs e)
{
WebReference.Service1SoapClient proxy = new
WebReference.Service1SoapClient();
this.DataContext =
proxy.CreateObject();
}
The output of the CreateObject
method is of WebContent type and is set as DataContext for the Window object.
Once we do this, all accessible properties of the type are available for binding
to different elements in the window.
Now modify the XAML code with bindings
to the properties as below:
<TextBlock Grid.Column="0" Grid.Row="0"
FontWeight="Bold" Text="Greeting Message:"/>
<TextBlock Grid.Column="1" Grid.Row="0"
FontWeight="Bold" Text="{Binding Path=GreetingString}" Foreground="Blue"
/>
<TextBlock Grid.Column="0" Grid.Row="1"
FontWeight="Bold" Text="List of Names: "/>
<ListBox Grid.Column="1" Grid.Row="1"
Margin="0,3,0,0" ItemsSource="{Binding Path=Names}" Foreground="Blue"
/>
Build the WPF window project and press
F5 to run it. The output is as shown below:
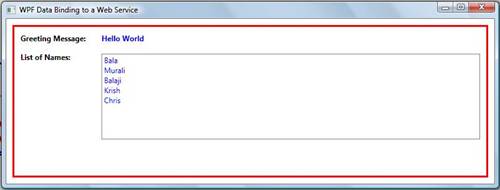
Other Options:
Yes, it is simple to bind the results
of a Web Service method to a WPF element. Instead of Data Binding technique used
in the article, you could also simply add a service reference to a web service,
create a proxy object and directly assign it to the controls' property to the
return value of a web method as given below.Assume that the web service has a
method named "GetName" that returns a string value.
this.TextBox1.Text =
proxy.GetName();
Alternatively, if a Web service is
available and accessible in the local network, you may add a reference to the
assembly and use the ObjectDataProvider to perform binding to the web method as
below.
First add the following markup in the
Window tag for defining namespace and reference the assembly.
xmlns:Remote="clr-namespace:MyWebService;assembly=MyWebService"
Then, declare an ObjectDataProvider and
bind it to a Label control
|