This approach will provide a better user experience when
our input forms have large number of input controls. Once this is in place, the
users can identify the input control very easily that have the wrong data in a
big input forms since it is highlighted. Something like below,
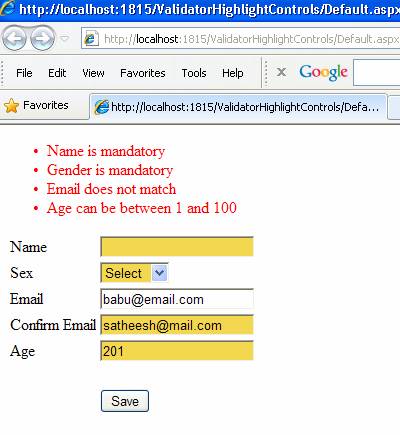
To do this, I will use jQuery library for the client
side scripting in this article. I assume you have created a new asp.net project
in your visual studio to understand this article.
By default, when we use Asp.Net Validator controls the
framework will emit a JavaScript array called Page_Validators in the output HTML
populated with the list of Validator controls on the page. See Listing 1 below.
This array will be in turn consumed by the inbuilt validation script emitted by
Asp.Net (through WebResource.axd handler, you can see it in view source) along
the page HTML for performing the validations on the client side.
Listing 1 – Validator Array
<script type="text/javascript">
//<![CDATA[
var Page_ValidationSummaries = new
Array(document.getElementById("ValidationSummary1"));
var Page_Validators = new
Array(document.getElementById("RequiredFieldValidator1"),
document.getElementById("RequiredFieldValidator2"),
document.getElementById("RegularExpressionValidator1"),
document.getElementById("CompareValidator1"),
document.getElementById("RangeValidator1"));
//]]>
</script>
Along with the above array, the Asp.Net also emits some
data associated with every validation controls in the form of JavaScript object
and let the inbuilt validation script consume it for performing the validations.
See below,
Listing 2 – Validator Data
<script type="text/javascript">
//<![CDATA[
var RequiredFieldValidator1 = document.all ?
document.all["RequiredFieldValidator1"] :
document.getElementById("RequiredFieldValidator1");
RequiredFieldValidator1.controltovalidate = "txtName";
RequiredFieldValidator1.errormessage = "Name is
mandatory";
RequiredFieldValidator1.display = "None";
RequiredFieldValidator1.evaluationfunction =
"RequiredFieldValidatorEvaluateIsValid";
RequiredFieldValidator1.initialvalue = "";
var RequiredFieldValidator2 = document.all ?
document.all["RequiredFieldValidator2"] :
document.getElementById("RequiredFieldValidator2");
RequiredFieldValidator2.controltovalidate =
"ddlGender";
RequiredFieldValidator2.errormessage = "Gender is
mandatory";
RequiredFieldValidator2.display = "None";
RequiredFieldValidator2.evaluationfunction =
"RequiredFieldValidatorEvaluateIsValid";
RequiredFieldValidator2.initialvalue = "0";
……trimmed for brevity
//]]>
</script>
We will make use of this information and with the help
of jQuery let’s see how we can highlight the input control whenever the
validation fails.
If we take a closer look at the data emitted by the
Asp.Net framework in Listing 2, each validator control has a property called
controltovalidate that holds the associated input control and initialvalue that
holds the initial value the input control. The validator control will validate
the input based on the initialvalue set in the property, in other words, the
validation will fail if the initialvalue and value in input control matches for
required field validations. The rest of the properties seen in the above
listings are self explanatory. Apart from the above property each validator
control has a property called isvalid which will return true or false based on
the validation outcome. This property “isvalid” is the key of this article since
it holds the validation outcome for individual validations.
|