The implementation of AJAX enabled application is made
easy with the help of 2 new controls called UpdatePanel control and
ScriptManager control in ASP.Net AJAX framework. These are the two primary
controls that makes AJAX happen with very less effort. This article will help us
understand the usage of these two new controls by implementing a sample
application. After reading this article, the reader will have good understanding
on these controls and he can use this controls effectively to build AJAX
applications.
To make our understanding better, we will develop a
sample application and understand these controls precisely.
Pre-Requisites
For ASP.Net 2.0, ASP.Net AJAX is
available as ASP.NET AJAX
Extensions 1.0 for free download. For ASP.Net 3.5, AJAX functionality is
integrated in ASP.NET 3.5 and does not require any additional
downloads. Download and install the library on your development machine to
start developing ASP.Net AJAX applications.
Sample
We will build an application which displays Employees
details in a Gridview that has edit/update/delete feature. We can also include a
feature to insert new employee details in the same page. Refer the below figure.
The button “Clear Employee Display” will clear all the employee display in the
gridview. Since we are developing this application in AJAX, we will have a label
in left-hand top corner that displays the server time to know when the last postback to
server is happened.
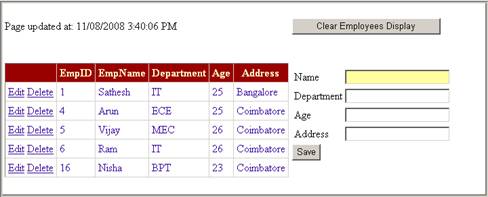
As I said earlier, the AJAX enabled application with
ASP.Net AJAX framework is done through the primary support of 2 new controls,
UpdatePanel and ScriptManager. Before moving to the actual implementation we
will have some knowledge about these controls.
ScriptManager Control
This is the control that manages and registers the
client script for aspx pages for asynchronous request/response processing. All
the Ajax controls require this control to be present on the page to communicate
asynchronously to the server. This control should be kept on top of the page
before any control for the AJAX to work.
UpdatePanel Control
Ø
This is the control that makes the partial page rendering
possible.
Ø
UpdatePanel control works together with ScriptManager control for
Asynchronous request/response processing.
Ø
By default, the controls that are added inside the UpdatePanel
control becomes Ajax enabled controls. All the server controls inside the
UpdatePanel control can post the panels content to server asynchronously and
refresh the panel’s content.
Ø
The child controls that causes asynchronous postback is called the
Triggers of UpdatePanel.
If we want to build an Ajax enabled application, our
target will be refreshing the part of page instead of whole page. Hence, we can
have more than one UpdatePanel control to refresh different part of the page
asynchronously. If we have more than one UpdatePanel control in a page, by
default the contents of all the UpdatePanel controls will be refreshed for any of
the asynchronous postbacks caused on the page i.e. even for the asynchronous
postback in other UpdatePanel controls. This is the default behavior of
UpdatePanel controls. There is a property called UpdateMode to control this
behavior. It takes an enumerator that accepts either “Always” or “Conditional”.
The default value will be “Always” and hence the above said behavior is
seen.
Syntax
<asp:UpdatePanel ID="UpdatePanel3"
runat="server">
<ContentTemplate>
//Controls go here
</ContentTemplate>
</asp:UpdatePanel>
With this information, we will start building our sample
application. We will have 3 UpdatePanel controls in our application, one for
GridView, another for Inserting New Row and one more for displaying last
postback time. We will have the “Clear Employee Display” button outside the
UpdatePanel and we will implement it as Ajax enabled control using ScriptManager
control.
Step 1
Drag an UpdatePanel control from the “Ajax Extensions”
tab of Visual studio 2005. Drag a SqlDataSource control and configure it. I have
used the database attached in App_Data in this sample. Configure Update/Delete
by clicking “Advanced” button and checking “Generate Insert, Update, and Delete
statements”. Refer the below figures for clear understanding.
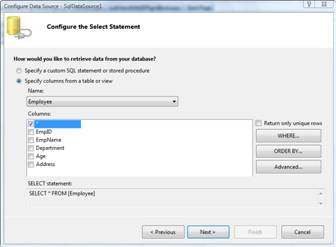
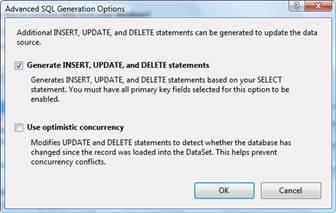
Step 2
Drag a GridView control and configure its DataSourceID
property to the SqlDataSource control’s ID. Check “Enable Editing” and “Enable
Deleting” option in the GridView[When we click smart tag we will get this option].
Execute the webpage and you can see edit/update/delete
working without postback. Next, we will add insert functionality as seen in the
sample application's output figure.
Insert New Row
Step 1
Drag an UpdatePanel control. Drag textbox for name,
department, age and address and a button for save.
<asp:UpdatePanel ID="UpdatePanel2" runat="server"
UpdateMode="Conditional">
<ContentTemplate>
<table>
<tr>
<td style="width:
100px">
Name
</td>
<td style="width:
100px">
<asp:TextBox
ID="txtEmpName" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td style="width:
100px">
Department
</td>
<td style="width:
100px">
<asp:TextBox
ID="txtdept" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td style="width:
100px">
Age
</td>
<td style="width:
100px">
<asp:TextBox ID="txtAge"
runat="server"></asp:TextBox></td>
</tr>
<tr>
<td style="width:
100px">
Address
</td>
<td style="width:
100px">
<asp:TextBox
ID="txtAddress" runat="server"></asp:TextBox></td>
</tr>
</table>
<asp:Button ID="btnSave" runat="server"
Text="Save" OnClick="btnSave_Click" />
</ContentTemplate>
</asp:UpdatePanel>
Step 2
On btnSave click,
SqlDataSource1.InsertCommand = "INSERT INTO
[Employee] ([EmpName], [Department], [Age], [Address]) VALUES ('" +
txtEmpName.Text + "', '" + txtdept.Text + "', '" + txtAge.Text + "', '" +
txtAddress.Text + "')";
SqlDataSource1.Insert();
When we execute the page, we can see insert happening
without postback. As I told before, any postback to the server caused by any
controls on the page will update the contents of other UpdatePanels on the page
by default. Hence, the newly added row will be updated on the GridView.
|