The client script can make a request to a web method and pass data as
input parameters to the method; and data can also be sent back to the client
browser from the server.
To enable your application to call ASP.NET Web services
by using client script, the server asynchronous communication layer
automatically generates JavaScript proxy classes. A proxy class is generated for
each Web service for which an <asp:ServiceReference> element is included
under the <asp:ScriptManager> control in the page.
<asp:ScriptManager runat="server"
ID="scriptManagerId">
<Scripts>
<asp:ScriptReference
Path="CallWebServiceMethods.js" />
</Scripts>
<Services>
<asp:ServiceReference
Path="WebService.asmx" />
</Services>
<asp:ScriptManager runat="server"
ID="scriptManagerId">
This proxy class is downloaded to the browser at page
load time and provides a client object that represents the exposed methods of a
Web service. To call a method of the Web service, you call the corresponding
method of the generated JavaScript proxy class. The proxy class in turn
communicates with the Web service. The requests are made asynchronously, through
the XMLHTTP object of the browser.
<asp:ScriptReference> element is specified to
register a javascript file that is to be used in a web page. Only after
registering the CallWebServiceMethod.js file, you can call methods on it.
Calling a Web service method from script is
asynchronous. To get a return value or to determine when the request has
returned, you must provide a succeeded callback function. The callback function
is invoked when the request has finished successfully, and it contains the
return value (if any) from the Web method call. You can also provide a failed
callback function to handle errors. Additionally, you can pass user context
information to use in the callback functions.
JSON – JavaScript Object Notation is the
default serialization format using which data transformation takes place between
client-server requests. You may disable all currently enabled protocols like
HTTP-GET, HTTP-POST, and even XML-SOAP formatting used in the earlier forms of
web services. The following settings in the Web.Config file just do the
same.
<system.web>
<webServices>
<protocols>
<clear/>
</protocols>
</webServices>
</system.web>
The figure below shows in detail the different layers
that lays on both the client and server sides. A request to a web service method
passes through these layers. You can see how a method is called on to one of the
available proxy object and the web request is handled by an XmlHttp object on
the client browser side. On the server side, your request is handled by an HTTP
handler as usual and sent for XML/JSON serialization.
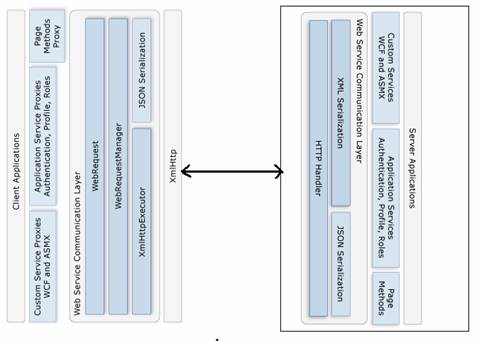
Example: Consuming WebService methods in AJAX
Consuming web service methods in AJAX involves two
steps. The first step is that there is a slight change in the way you create and
define web services. Making calls using client script from a page to the service
methods will be the second step.
Step 1: Creating a web service
In the System.Web.Scripts.Services namespace, you may
find an attribute class “ScriptSrvice” and this need to be applied to a
webservice class so that the web service methods can be invoked from the client
script. This would enable the proxy generation script to generate a proxy object
that corresponds to the webservice class.
Similarly, in the same namespace, you may find another
attribute class “ScriptMethod” and upon applying this attribute to a web
method you can specify which HTTP verb is used to invoke a method and the format
of the response. This attribute has three parameters described as below:
UseHttpGet: Setting it to true will
invoke the method using the HTTP GET command. The default is
false.
ResponseFormat: Specifies whether the
response will be serialized as JSON or as XML. The default is
Json.
XmlSerializeString: Specifies whether
all return types, including string types, are serialized as XML. The value of
the XmlSerializeString property is ignored when the response is serialized as
JSON.
Ok... Now, create a new Web Site using the ASP.NET Web
Service template in Visual Studio 2008 and modify the webservice class as
below.
using System.Web.Script.Services;
namespace AjaxWebService
{
[WebService(Namespace =
"http://localhost:49232/AjaxWebService/")]
[WebServiceBinding(ConformsTo =
WsiProfiles.BasicProfile1_1)]
[ScriptService]
public class Service :
System.Web.Services.WebService
{
string myXmlData = @"<?xml version=""1.0""
encoding=""utf-8"" ?>
<Book>
<Title>Programming ASP.NET 3.5,
AJAX and Web Services</Title>
</Book>";
// This method uses JSON response formatting
[ScriptMethod(ResponseFormat =
ResponseFormat.Json)]
[WebMethod]
public string getNextBackupDate(int months)
{
return
DateTime.Now.AddMonths(months).ToShortDateString();
}
// This method uses XML response formatting
[ScriptMethod(ResponseFormat =
ResponseFormat.Xml)]
[WebMethod]
public string Greetings(string name)
public XmlDocument GetBookTitle()
{
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.LoadXml(myXmlData);
return xmlDoc;
}
// This method uses HTTP-GET protocol to call it
[ScriptMethod(UseHttpGet = true)]
[WebMethod]
public string HelloWorld()
{
return "Hello, world";
}
}
}
Note: The web service created with ScriptService enabled as
above will not be tested on the browser by default. You need to modify the
settings in the Web.Config file as below to test the above
webservice.
<webServices>
<protocols>
<add name="HttpGet"/>
<add name="HttpPost"/>
</protocols>
</webServices>
|