Using UpdateProgress Control
<asp:UpdateProgress ID="UpdateProgress1"
runat="server">
<ProgressTemplate>
<DIV id="IMGDIV" align="center" valign="middle"
runat="server" style="position: absolute;left: 35%;top:
25%;visibility:visible;vertical-align:middle;border-style:inset;border-color:black;background-color:#c8d1d4;">
<asp:Image ID="Image1"
runat="server" ImageUrl="~/icon_inprogress.gif" />
</DIV>
</ProgressTemplate>
</asp:UpdateProgress>
When we click on any operation on the page, the image
will be displayed as an indication to the users for progressing.
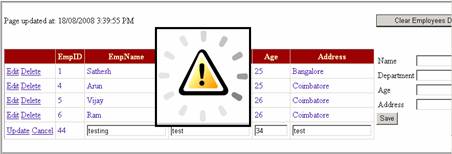
The image which is displayed in the page is inside the
UpdateProgress control. Moving forward, we will some advanced usages of
UpdateProgress control in this article.
Displaying a Cancel button to Cancel the Asynchronous
Postback with UpdateProgress control
When notifying about the progress of the asynchronous
request to the user, it will be good if we have option to cancel the request.
This can be done through the UpdateProgress control and abortPostBack() method
of PageRequestManager class. The below JavaScript function will help us do
that.
function CancelPostBack() {
var objMan =
Sys.WebForms.PageRequestManager.getInstance();
if (objMan.get_isInAsyncPostBack())
objMan.abortPostBack();
}
To call this function, include a HTML button in the
UpdateProgress control and call the above method in onclick event.
<asp:UpdateProgress ID="UpdateProgress1"
runat="server">
<ProgressTemplate>
<DIV id="IMGDIV" align="center"
valign="middle" runat="server" style="position: absolute;left: 35%;top:
25%;visibility:visible;vertical-align:middle;border-style:inset;border-color:black;background-color:White">
<img src=icon_inprogress.gif
/><br />
<input type="button"
onclick="CancelPostBack()" value="Cancel" />
</DIV>
</ProgressTemplate>
</asp:UpdateProgress>
If we execute the page, we can see the cancel button
appearing which will cancel the postback and the results in no refresh of
contents on the update panels.
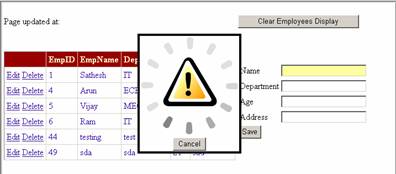
Drawback of the above approach
In the above approach, the abortPostBack()
function will cancel only the update/refresh of UpdatePanel control and thus,
whatever serverside processing is initiated will not be aborted. Hence, the
server side processing will move forward and completes its execution, but the
actual results will not be updated in the update panel.
It is advisable to use this functionality when there are
no persisting operations like database operations, etc done on the server. It
can be used to cancel some simple operations like loading controls, etc
dynamically depending on some server side business rules.
Associating a UpdateProgress Control to a UpdatePanel
If we see the implementation in previous sections, the
UpdateProgress control will be enabled for every asynchronous postback
originated from any where in the page. It is possible to limit to the
UpdateProgress control to be enabled only for the asynchronous postback
originated from a particular UpdatePanel control. To do this, there is a
property called AssociatedUpdatePanelID which accepts an UpdatePanel
control ID present on the page. Setting this Property will enable the
UpdateProgress control only for the postback originated from the particular
UpdatePanel.
<asp:UpdateProgress ID="UpdateProgress1" runat="server"
AssociatedUpdatePanelID="UpdatePanel1">
<ProgressTemplate>
<DIV id="IMGDIV" align="center"
valign="middle" runat="server" style="position: absolute;left: 35%;top:
25%;visibility:visible;vertical-align:middle;border-style:inset;border-color:black;background-color:White">
<img src=icon_inprogress.gif
/><br />
<input type="button"
onclick="CancelPostBack()" value="Cancel" />
</DIV>
</ProgressTemplate>
</asp:UpdateProgress>
The drawback of this approach is, it will not enable the
UpdateProgress control for a postback caused by an external trigger of the
UpdatePanel. It is by design of this property AssociatedUpdatePanelID.
The Implementation of enabling the UpdateProgress control is done by moving
through the control hierarchy in UpdatePanel control identified via
AssociatedUpdatePanelID to find a match for the control that causes the
actual postback. Since, the external triggers will not be present in the
hierarchy it will not enable the UpdateProgress control.
This drawback can be prevented by injecting a simple
JavaScript code. For example, if we have a external trigger (btnClear) to a
UpdatePanel control(UpdatePanel1). We can enable the UpdateProgress control for
the postback caused by btnClear manually by the following JavaScript
function.
<script type="text/JavaScript"
language="JavaScript">
function pageLoad()
{
var manager =
Sys.WebForms.PageRequestManager.getInstance();
manager.add_endRequest(endRequest);
manager.add_beginRequest(OnBeginRequest);
}
function OnBeginRequest(sender, args)
{
var postBackElement = args.get_postBackElement();
if (postBackElement.id == 'btnClear')
{
$get('UpdateProgress1').style.display = "block";
}
}
</script>
The UpdateProgress control will be rendered as a DIV tag
and can be enabled by setting its display CSS property to block by
hooking in from OnBeginRequest() event of PageRequestManager object. Through
this approach, it is possible to enable different UpdateProgress control for
different postback element on the page.
|