Introduction
WPF, an acronym for Windows Presentation
Foundation is a subsystem of class libraries for WinFX and it enables the
user to get a richer experience bringing together UI, Documents, media etc. A
XAML (Extensible Application Markup Language) file which is at the heart of a
WPF project can be created in several ways that includes the Notepad text
editor, the Expression Blend which requires another download from Microsoft, but
may not provide a easy to use XAML file to use in VS, and the Visual Studio
editions except the express edition. XAML is presently specific to windows
platform and is a XML formatting language and not an application programming
interface. I will be mostly showing how to get some hands-on experience with a
WPF project using the Visual Studio 2005 interface and the template files that
you may access with the Windows SDK installed.
Creating a WPF Project
From File | New | Project click open the New
Project window as shown in the next figure. Click on Visual Basic and expand
its contents. Under .NET 3.0 FrameWork (It is assumed that you have installed
NET 3.0 Framework) choose the Windows Application (WPF).
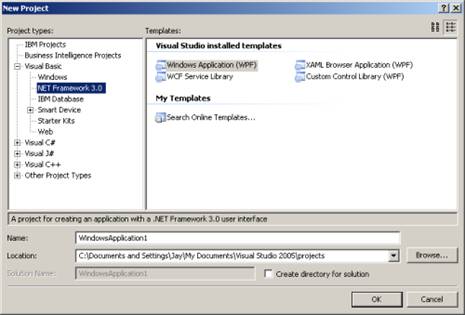
Now highlight the Windows Application (WPF) and
change the name of the application to some name of your choice. For this article
it is changed to AppWPF. Click on the OK button after typing a
name of your choice. This creates the necessary file/folders for the application
as shown in the next figure.
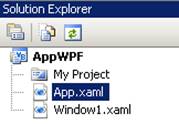
There are two XAML files created in the project. The
App.xaml and the Windows1.xaml file. Delete the
Windows1.xaml and add a new item as shown with the name
BasicControls.xaml.
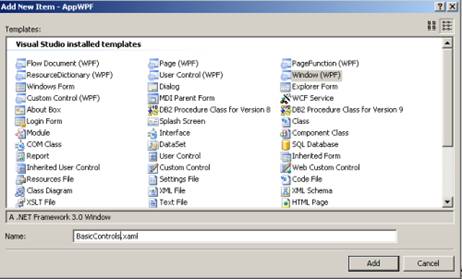
With this new item added you may need to change the
App.xaml file as shown below.
<Application x:Class="App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
StartupUri="BasicControls.xaml">
<Application.Resources>
</Application.Resources> </Application>
The StartupUri has been changed from the original
Windows1.xaml to BasicControls.xaml. With this change made you can now display
the BasicControls.xaml file together with its design as shown in the next
figure.
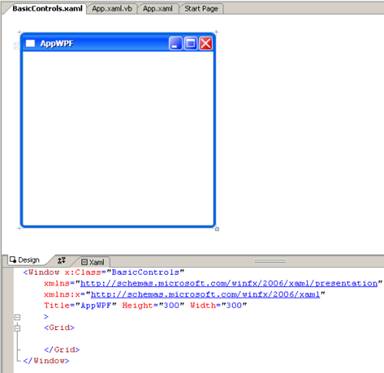
This represents a 300 X 300 window which can be used as
a container for other controls. You also notice the reference to the namespaces
that are required and the XML syntax with the attribute of the project for the
window.
Placing Controls on the Window
Placing Controls automatically creates XAML
code.
Placing controls on this window is as easy as dragging
from the Tools and dropping on to this window. The next picture shows a
button and a textbox dragged and dropped onto this window.
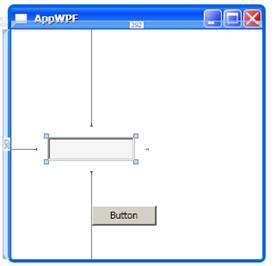
The necessary code for these controls gets automatically
added as the controls are placed. After the two controls are added, the xaml
file gets changed as shown. The Button and Textbox properties are
the defaults which may be modified as will be seen later in the article.
<Window x:Class="BasicControls"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="AppWPF"
Height="300" Width="300" > <Grid> <Button
Height="23" Margin="94,0,123,39" Name="Button1"
VerticalAlignment="Bottom">Button</Button> <TextBox
HorizontalAlignment="Left" Margin="43, 126, 0,115"
Name="TextBox1" Width="100"></TextBox>
</Grid> </Window>
Adding code automatically updates the window design.
Inserting a declarative code into the BasicControls.xaml
file will automatically add the control defined by that code to the design
window.
Add this code to the xaml file after as shown in the
next paragraph.
As soon as you type "<", the intellisense gets fired
up and you will see a drop-down list of items that you can insert as shown in
the next figure.
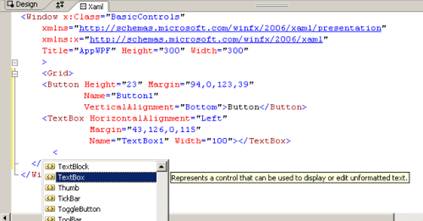
Now you click on the Textbox (or whatever else you wish
to place). This adds to the xaml file. Now to the opening tag of the textbox,
you add a name attribute and call it TextBox2. Intellisense is also used
in adding attributes as you will get a context sensitive listing of attributes
for the chosen control. Also add other attributes such as width, height,
alignment etc. With the code added as shown in the next paragraph you will see
that the design pane has a new textbox as shown in the next figure.
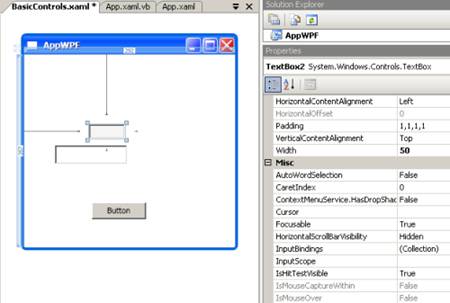
<Textbox Name="TextBox2" Height="20"
Margin="89.5,96.5,0,0" VerticalAlignment="Top"
HorizontalAlignment="Left" Width="50"></TextBox>
The property window for the TextBox2 shown can
also be used to make changes. You can also move, or adjust the dimensions of the
controls using the mouse. The various controls provide a very rich interface for
the designer in manipulating the controls.
|