In this article, we will
overcome this difficulty and provide an edit update feature similar to GridView
control using the powerful jQuery library and Ajax.
Refer the below figure to understand
better.
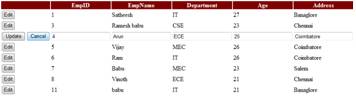
Steps
1. Open Visual Studio 2008 IDE.
2. Using a language of your choice, create a new website with appropriate name.
3. Drag a Repeater control from the data tab of the Visual
Studio.
4. You can add a Sql server Express database in App_Data Folder and
create a table called Employee with all the necessary fields. Enter some sample
data to display in the Repeater control.
5. Specify the HeaderTemplate, ItemTemplate and FooterTemplate to
display the employee data in tabular format. Bind the Repeater control from
codebehind by fetching employee detail from the express database we have created
in App_Data folder.
Refer the
below code,
ASPX
<asp:Repeater ID="rpEmployee"
runat="server">
<HeaderTemplate>
<table border="0"
ID="tblEmployee">
<tr id="Tr2" runat="server"
style="">
<th id="Th1">
</th>
<th id="Th2">
EmpID</th>
<th id="Th3">
EmpName</th>
<th id="Th4">
Department</th>
<th id="Th5">
Age</th>
<th id="Th6">
Address</th>
</tr>
</HeaderTemplate>
<ItemTemplate>
<tr ID='<%# Eval("EmpID")
%>'>
<td>
<input
type="button" value="Edit"/>
<input
type="button" value="Update" style="display:none" />
<input
type="button" value="Cancel" style="display:none" />
</td>
<td>
<%#
Eval("EmpID") %>
</td>
<td>
<%#
Eval("EmpName") %>
</td>
<td>
<%#
Eval("Department") %>
</td>
<td>
<%# Eval("Age")
%>
</td>
<td>
<%#
Eval("Address") %>
</td>
</tr>
</ItemTemplate>
<FooterTemplate>
</table>
</FooterTemplate>
</asp:Repeater>
CodeBehind
protected void Page_Load(object sender,
EventArgs e)
{
rpEmployee.DataSource =
GetEmployee("Select * from Employee");
rpEmployee.DataBind();
}
public DataTable GetEmployee(string
query)
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["DatabaseConnectionString"].ConnectionString);
SqlDataAdapter ada = new
SqlDataAdapter(query, con);
DataTable dtEmp = new
DataTable();
ada.Fill(dtEmp);
return dtEmp;
}
Web.Config
<connectionStrings>
<add name="DatabaseConnectionString"
connectionString="Data
Source=.\SQLEXPRESS;AttachDbFilename=|DataDirectory|\Database.mdf;Integrated
Security=True;Connect Timeout=30;User Instance=True"
providerName="System.Data.SqlClient"
/>
</connectionStrings>
Execute the page and you can see the
employee details in tabular view with an edit button.
Next, we will make the Repeater
control’s row editable similar to the inbuilt edit feature of GridView control
using jQuery library.
Providing Edit Update
Feature
If you are not familiar with jQuery
library, I encourage you to read the following FAQ’s to have a basic
understanding on the library.
What
is jQuery? How to use it in ASP.Net Pages?
How
to enable jQuery intellisense in Visual Studio 2008?
How
to use jQuery intellisense in an external javascript file?
When the user clicks the edit button,
we will hide the edit button and enable update & cancel button for that row.
Just like GridView control, we will also populate the table cell value of the
editing row in a textbox control to edit. After editing the value and on
clicking Update button, we call a page method called UpdateEmployee() to update
the employee details in the database using the jQuery’s ajax() method.
First, we will develop our page method
UpdateEmployee() in our codebehind to update the employee details that is passed
to it from jQuery’s ajax method.
[WebMethod]
public static string
UpdateEmployee(string empid,string name,string dept,string age,string address)
{
string UpdateQuery = "UPDATE
[Employee] SET [EmpName] = @name, [Department] = @dept, [Age] = @age, [Address]
= @address WHERE [EmpID] = @empid";
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["DatabaseConnectionString"].ConnectionString);
con.Open();
SqlCommand com = new
SqlCommand(UpdateQuery, con);
com.Parameters.Add("@name",
SqlDbType.VarChar, 50).Value = name;
com.Parameters.Add("@dept",
SqlDbType.VarChar, 50).Value = dept;
com.Parameters.Add("@age",
SqlDbType.Int, 4).Value = age;
com.Parameters.Add("@address",
SqlDbType.VarChar, 50).Value = address;
com.Parameters.Add("@empid",
SqlDbType.Int, 4).Value = empid;
int n =
com.ExecuteNonQuery();
con.Close();
return n.ToString();
}
|