GridView control
DataGrid control in ASP.Net 1.x is replaced by this
control in ASP.Net 2.0 release and it is one of the most widely used controls.
Gridview control can be used in those scenarios where we need to display data in
tabular format. Refer the below figure,
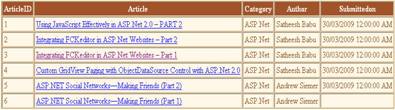
Using this control is very easy when compared to other
databound controls to display data. Just assigning the DataSource property to a
DataTable and calling DataBind() method will render the data. The main
disadvantage of GridView control is, it does not have the flexibility to display
data in any other format except table.
Gridview control will actually render the data in
<Table> tag. Each column will be rendered as separate TD which makes it
not possible to display data in any other format. Not to mention only its
disadvantages, it is the only databound control which has inbuilt edit, update,
delete, sort, paging data functionality. We can enable these functionalities by
setting the properties of GridView without even writing a single line of code.
How to use GridView control?
As I said earlier, just assigning the DataSource
property to a DataTable object and calling DataBind() method will render the
data. Else, we can assign the DataSourceID property to the datasource id will
display the data.
We can also specify the columns ourselves using GridView
control where we can provide custom styles to the GridView control like the
above figure. Gridview control has number of inbuilt column types that includes
the column types from DataGrid control and additional new column types like
Button field, Checkbox field, Hyperlink field, Image field which can be used.
Set the AutoGenerateColumns property to false when you are defining the columns yourself.
Refer the below code,
<asp:GridView ID="gvArticles" runat="server"
BackColor="#DEBA84" EnableViewState="false" BorderColor="#DEBA84"
BorderStyle="None" BorderWidth="1px"
CellPadding="3" CellSpacing="2" AutoGenerateColumns="False"
DataKeyNames="ArticleID" DataSourceID="SqlDataSource1">
<FooterStyle BackColor="#F7DFB5"
ForeColor="#8C4510" />
<RowStyle BackColor="#FFF7E7"
ForeColor="#8C4510" />
<SelectedRowStyle BackColor="#738A9C"
Font-Bold="True" ForeColor="White" />
<PagerStyle ForeColor="#8C4510"
HorizontalAlign="Center" />
<HeaderStyle BackColor="#A55129"
Font-Bold="True" ForeColor="White" />
<Columns>
<asp:BoundField DataField="ArticleID"
HeaderText="ArticleID" InsertVisible="False"
ReadOnly="True" SortExpression="ArticleID"
/>
<asp:HyperLinkField
HeaderText="Article" DataTextField="Title" DataNavigateUrlFields="URL"
/>
<asp:BoundField DataField="Category"
HeaderText="Category" SortExpression="Category" />
<asp:BoundField DataField="Author"
HeaderText="Author" SortExpression="Author" />
<asp:BoundField DataField="Submittedon"
HeaderText="Submittedon" SortExpression="Submittedon" />
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>"
SelectCommand="SELECT * FROM
[Articles]"></asp:SqlDataSource>
I have used SqlDataSource control to bind the GridView
in the above code.
Using this control to just display data is really heavy
because it loads the viewstate with state informations to provide the inbuilt functionalities like sort,
paging, etc. Disable the viewstate in this case if you are not using any of the
inbuilt functionalities to improve the performance.
Read the GridView articles posted on CodeDigest
here,
Custom
GridView Paging with ObjectDataSource Control with ASP.Net 2.0
Building
Sharepoint List Style GridView with Ajax Control Toolkit
How
to format DateTime in GridView BoundColumn and TemplateColumn?
GridView
with CheckBox – Select All and Highlight Selected Row
GridView
with RadioButton – Select One at a Time
GridView
with Thumbnail Images – Part 3
GridView
with Thumbnail Images – Part 2
GridView
with Thumbnail Images – Part 1
Useful
GridView Tips
GridView
with Image
DataList Control
DataList is one another databound control that can be
used to display data. Displaying data with DataList control is not as easy as
GridView control. Apart from specifying the datasource and calling DataBind()
method, we need to specify the data items display using ItemTemplate and
DataBinding expressions(Eval and Bind). Unlike GridView, providing edit, delete,
sort and paging functionalities with this control involves a bit of coding effort and
not easy as GridView control.
This control is bit flexible enough to display data in
various formats, most importantly in flow and tiled format. DataList control
gives the flexibility to the developers to decide how to display the rows of
database information as opposed to GridView control (In GridView, all the
columns are displayed in TD). Refer the below figure,
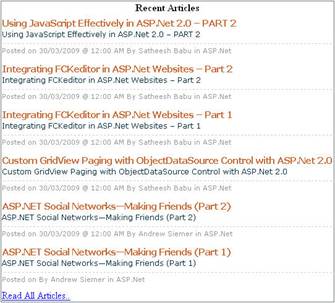
<asp:DataList ID="dlArtilces" BorderStyle="Solid"
DataSourceID="SqlDataSource1" BorderColor="#626e6e" BorderWidth="1px"
runat="server">
<HeaderTemplate>
<div>
<div style="Text-align:center;">
<b>Recent
Articles</b></div>
</div>
</HeaderTemplate>
<ItemTemplate>
<div class="Post">
<div
class="PostTitle"><asp:HyperLink ID="TitleLink" runat="server"
Text='<%# Eval("Title") %>' NavigateUrl='<%# Eval("URL")
%>'/></div>
<div
class="PostSubtitle"><asp:Label ID="SubtitleLabel" runat="server"
Text='<%# Eval("Title") %>' /></div>
<div class="PostInfo">
Posted on <asp:Label
ID="DatePostedLabel" runat="server" Text='<%# Eval("Submittedon", "{0:d} @
{0:t}") %>' />
By <asp:Label ID="lblAuthor"
runat="server" Text='<%# Eval("Author") %>' /></a>
in <%# Eval("Category")
%>
</div>
</div>
</ItemTemplate>
<FooterTemplate>
<div>
<div>
<a href="Articles.aspx">Read All
Articles..</a></div>
</FooterTemplate>
</asp:DataList>
<asp:SqlDataSource ID="SqlDataSource1"
runat="server" ConnectionString="<%$ ConnectionStrings:ConnectionString
%>"
SelectCommand="SELECT * FROM
[Articles]"></asp:SqlDataSource>
The above code uses SqlDataSource control to interact
with the database.
DataList control will populate the whole data inside a
HTML table by default, where each row is enclosed in a TD. You can check the view source
and understand this. This control also has the capability to enclose the display
of data in DIV tag instead of Table. To do this, set RepeatLayout property to
"Flow".
In DataList control, the rows of data are repeated in
the layout specified in the ItemTemplate. This control by default will repeat
the rows in Vertical. We can also change to horizontal display by setting the
property RepeatDirection to Horizontal.
Read the DataList articles posted on CodeDigest
here,
Creating
Dynamic DataList control in C#/ASP.Net
|