Something like below,
Figure 1 - Select multiple files
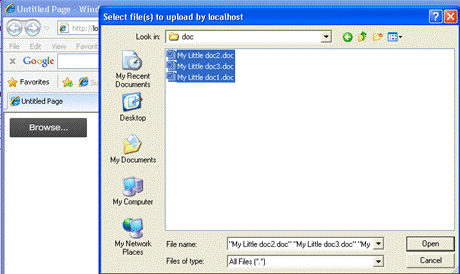
Figure 2 - Upload multiple files
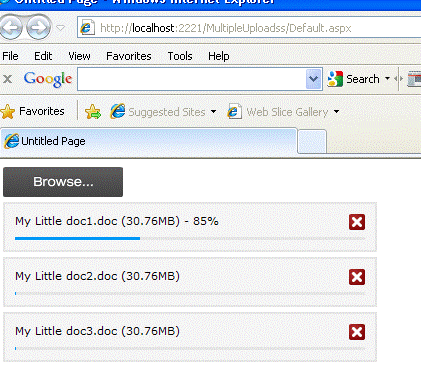
How to do this?
Well, this can be done very easily by using a jQuery
file upload plug-in called Uploadify
which uses flash for rich Ajax like interface. Basically, when you use
this plug-in you will not see page refresh when uploading files to server. The
next section will help us to implement the above system in a step by step
approach.
Steps
1.
Create a sample web application project in your Visual Studio 2008.
In this article, i have used C# as the language of my choice.
2. Download the latest version of
jQuery library from the official site(http://www.jquery.com))and integrate into your project. Read the
following Faq’s to integrate jQuery library into your project.
What is
jQuery? How to use it in ASP.Net Pages?
How to
enable jQuery intellisense in Visual Studio 2008?
How to use
jQuery intellisense in an external javascript file?
Create a folder
called _scripts in in your project and put the jQuery library inside
it.
3. Next, create a folder in server
and provide appropriate permission (Read, Write & Modify)to the ASP.Net
service account in order to save the uploaded files. You can also configure this
folder in AppSettings of the project so that it can be accessed from code. I
have hard coded the path in this article for simplicity.
4. Download the latest Uploadify
plug-in from here. The latest
version as of this writing is v2.1.4. Unzip the folder and copy the below files
into _scripts directory in your project.
Ø
jquery.uploadify.v2.1.4.min.js
Ø
swfobject.js
Ø
uploadify.css
Ø
uploadify.swf
Ø
cancel.png
5. The Uploadify plug-in requires
an aspx page or an HttpHandler which can be called to upload the selected files
in the server. Hence, include a new aspx page or ashx handler in your project (
I have used aspx page called FileUploads.aspx.) This page will have the code to
upload the incoming file to the server folder. Refer the code below,
public partial class FileUploads : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs
e)
{
HttpPostedFile uploads =
Request.Files["FileData"];
string file =
System.IO.Path.GetFileName(uploads.FileName);
try
{
uploads.SaveAs("D:\\UploadedUserFiles\\" +
file);
}
catch
{
//exception handling code
}
}
}
6. Now, open your Default.aspx
page to design our main interface which the user can use to upload the files
which will use jQuery and Uploadify plug-in. Refer the code below,
Ajax like multiple file upload using Uploadify jQuery
plug-in
<head runat="server">
<link href="_scripts/uploadify.css" rel="stylesheet"
type="text/css" />
<script type="text/javascript"
src="_scripts/jquery-1.4.4.min.js"></script>
<script type="text/javascript"
src="_scripts/swfobject.js"></script>
<script type="text/javascript"
src="_scripts/jquery.uploadify.v2.1.4.min.js"></script>
<title>Untitled Page</title>
<script type="text/javascript">
$(document).ready(function() {
$('#fuFiles').uploadify({
'uploader':
'_scripts/uploadify.swf',
'script': 'FileUploads.aspx',
'cancelImg':
'_scripts/cancel.png',
'auto': 'true',
'multi': 'true',
'buttonText': 'Browse...',
'queueSizeLimit': 3
});
});
</script>
</head>
<body>
<form id="form1" runat="server">
<div id="fuFiles"></div>
</form>
</body>
</html>
|