Enabling Back button using Ajax History in ASP.Net
AJAX
Let’s say we have a requirement we need to display the
list of the employees with the page size 5 and the user wants paging to be done
using ajax and maintain the ajax history.
1. Take a GridView and bind it
with data from sql server and implement the paging. The markup looks as
below.
<asp:GridView
ID="GridView1" runat="server"
AutoGenerateColumns="False" Width="631px" AllowPaging="True"
onpageindexchanged="GridView1_PageIndexChanged"
onpageindexchanging="GridView1_PageIndexChanging"
PageSize="5" CellPadding="4"
ForeColor="#333333" GridLines="None">
<RowStyle
BackColor="#EFF3FB" />
<Columns>
<asp:BoundField DataField="sno" HeaderText="Sno" />
<asp:BoundField DataField="empname" HeaderText="EmpName" />
</Columns>
<FooterStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#2461BF" ForeColor="White" HorizontalAlign="Center" />
<SelectedRowStyle
BackColor="#D1DDF1" Font-Bold="True" ForeColor="#333333" />
<HeaderStyle
BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<EditRowStyle BackColor="#2461BF" />
<AlternatingRowStyle BackColor="White" />
</asp:GridView>
The Code is as below:
protected void
Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = GetDataSet();
GridView1.DataBind();
}
}
private DataSet GetDataSet()
{
SqlConnection con = new
SqlConnection("server=local;integrated
security=true;database=master");
DataSet ds = new DataSet();
SqlDataAdapter dta = new SqlDataAdapter("select
sno,empname from employees", con);
dta.Fill(ds, "test");
return ds;
}
protected void GridView1_PageIndexChanging(object
sender,
GridViewPageEventArgs e)
{
GridView1.PageIndex = e.NewPageIndex;
GridView1.DataSource = GetDataSet();
GridView1.DataBind();
}
protected void GridView1_PageIndexChanged(object sender, EventArgs e)
{
}
2. Now run the application and
check the output, user can click the paging buttons and traverse from page to
other pages of GridView records. Also the browser history is maintained and user
can move forward/backward using the backward and forward buttons. Check the
screenshot where the history is maintained
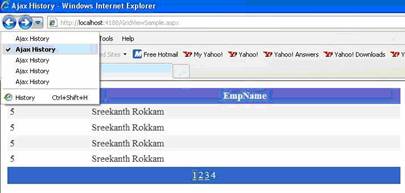
Note: Here Ajax History is the page title
given.
3. But our requirement is to make
paging requests as asynchronous post back. Let’s make the paging operations as
asynchronous operations using Updatepanel and Scriptmanager. Drag
the Scriptmanager to the page and place the GridView inside the
Updatepanel. The sample markup looks as below.
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<asp:UpdatePanel ID="UpdatePanel1"
runat="server">
<ContentTemplate>
<asp:GridView ID="GridView1" runat="server"
AutoGenerateColumns="False" Width="631px"
AllowPaging="True"
onpageindexchanged="GridView1_PageIndexChanged"
onpageindexchanging="GridView1_PageIndexChanging"
PageSize="5" CellPadding="4"
ForeColor="#333333" GridLines="None">
<RowStyle BackColor="#EFF3FB" />
<Columns>
<asp:BoundField DataField="sno"
HeaderText="Sno" />
<asp:BoundField DataField="empname"
HeaderText="EmpName" />
</Columns>
<FooterStyle BackColor="#507CD1" Font-Bold="True"
ForeColor="White" />
<PagerStyle BackColor="#2461BF" ForeColor="White"
HorizontalAlign="Center" />
<SelectedRowStyle BackColor="#D1DDF1"
Font-Bold="True"
ForeColor="#333333" />
<HeaderStyle BackColor="#507CD1" Font-Bold="True"
ForeColor="White" />
<EditRowStyle BackColor="#2461BF" />
<AlternatingRowStyle BackColor="White"
/>
</asp:GridView>
</ContentTemplate>
</asp:UpdatePanel>
4. Now run the application and
check the output by traversing page to page of GridView records. The postback is
made asynchronous and the browser history doesn’t work. Check the screenshot for
output.
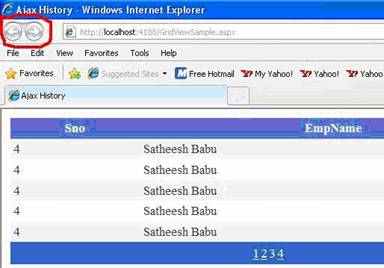
5. Now we need enable the Ajax
History, first change EnableHistory of Scriptmanager to “true”.
And then write the event onNavigate to Scriptmanager. The sample markup
looks as below.
<asp:ScriptManager ID="ScriptManager1" runat="server" EnableHistory="true" OnNavigate="ScriptManager1_Navigate">
</asp:ScriptManager>
6. In the code behind add the
AjaxHistory in the GridView PageIndex Changed event
protected
void GridView1_PageIndexChanged(object sender, EventArgs e)
{
string pageIndex = (sender as
GridView).PageIndex.ToString();
ScriptManager1.AddHistoryPoint("paging",
pageIndex);
}
On the ScriptManager Navigate Event, restore the
history point added and show the concerned page when clicked on the browser
history buttons
protected void
ScriptManager1_Navigate(object sender, HistoryEventArgs e)
{
if (e.State.Count <= 0)
{
GridView1.PageIndex = 0;
return;
}
string PageKey = e.State.AllKeys[0];
string pageIndex = String.Empty;
if (PageKey == "paging")
{
pageIndex = e.State[PageKey];
int iPageIndex =
Convert.ToInt32(pageIndex);
GridView1.PageIndex = iPageIndex;
}
GridView1.DataSource = GetDataSet();
GridView1.DataBind();
}
7. Now run the application and
check the output the browser back/forward buttons are enabled even for
asynchronous postbacks. Check the screenshot below after implementing Ajax
history.
We can also add Ajax HistoryPoints using client side
framework of scriptmanager too without using the server side utilities. Let’s
say user has another requirement like user can enter a student name on a textbox
and when a button is clicked, all the student details are fetched from server
using Pagemethods/webservices. Here are the steps to achieve the functionality
and implement Ajax history.
|