Transformation using GeneratedImage
control
To do transformation on the generated
image, there is an abstract called ImageTransform which can be implemented.
Transformation may be, adding watermark, adding copyright information on the
image etc.
ImageTransform abstract class
public abstract class
ImageTransform
{
protected
ImageTransform();
[Browsable(false)]
public virtual string UniqueString
{ get; }
public abstract Image
ProcessImage(Image image);
}
To understand this better, we will
implement a simple watermark with ImageTransform
class.
public class WaterMark :
ImageTransform
{
public WaterMark()
{
//
// TODO: Add constructor
logic here
//
}
public override System.Drawing.Image
ProcessImage(System.Drawing.Image img)
{
Graphics gra =
Graphics.FromImage(img);
gra.DrawString("www.microsoft.com", new Font("Verdana", 18), new
SolidBrush(Color.Green), img.Width / 2, img.Height / 2);
return img;
}
}
The above class will add a text www.microsoft.com in the middle of the
image.
Using the above transformation
class,
public class WaterMarkTransformatiom :
ImageHandler
{
public
WaterMarkTransformatiom()
{
}
public override ImageInfo
GenerateImage(NameValueCollection parameters) {
string imgurl =
HttpContext.Current.Server.MapPath(".")+"\\AutumnLeaves.jpg";
Bitmap img = new
Bitmap(imgurl);
WaterMark wmImage = new
WaterMark();
return new
ImageInfo(wmImage.ProcessImage(img));
}
}
In the above handler, We are reading a
image from file system and adding the water mark text by calling ProcessImage()
method of WaterMark transformation class. The output will be,
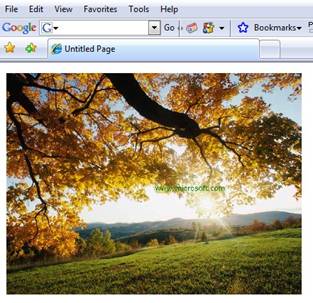
You can see the word www.microsoft.com
on the displayed image.
Adding Caching to the
control
The ImageHandler object has properties
to set both server and client cache. It also has property to set client cache
expiration time. This can be set in the constructor of handler that is
generating image.
The below code enables client and
server cache for the image handler that is generating image from
database,
public class ImageFromDB : ImageHandler
{
public ImageFromDB() {
// Set caching settings and add
image transformations here
this.EnableServerCache =
true;
this.EnableClientCache =
true;
}
public override ImageInfo
GenerateImage(NameValueCollection parameters) {
// Add image generation logic
here and return an instance of ImageInfo
string imageid =
parameters["ImID"].ToString();
SqlConnection connection = new
SqlConnection(ConfigurationManager.ConnectionStrings["connectionString"].ConnectionString);
connection.Open();
SqlCommand command = new
SqlCommand("select Image from Image where ImageID=" + imageid,
connection);
SqlDataReader dr =
command.ExecuteReader();
dr.Read();
this.ImageTransforms.Add(new
WaterMark());
return new
ImageInfo((Byte[])dr[0]);
}
}
|