Tracking Advertisement Using AdRotator
control
To track the advertisement in terms of
number of clicks or hits, we need to pass the user to the destination page (ad’s
landing page) through a proxy webpage or an HttpHandler. This proxy can then
update your click into the database and will redirect the user to target page.
Below is the database design which we
can use.
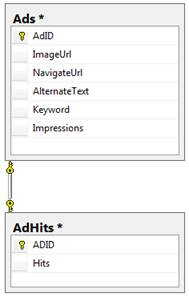
Drag an AdRotator control and a
SqlDataSource control from your visual studio toolbar. Bind the AdRotator
control through DataSourceID property after configuring the datasource control.
As i said before, we need to create a
proxy which can record the clicks and redirects the user to target page. We will
use HttpHandler to do this. Include a new HttpHandler to your solution through
“Add new Item..” dialog box, name it as AdHandler.ashx.
Refer the below code,
public class AdHandler :
IHttpHandler
{
public void
ProcessRequest(HttpContext context)
{
string url =
context.Request.QueryString["AdUrl"];
try
{
AddHit(url);
}
catch
{
}
finally
{
context.Response.Redirect(url);
}
}
public int AddHit(string
url)
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings ["ConnectionString"].ConnectionString);
con.Open();
SqlCommand com = new
SqlCommand("AddHit", con);
com.CommandType =
System.Data.CommandType.StoredProcedure;
com.Parameters.Add(new
SqlParameter("@url",url));
int res =
com.ExecuteNonQuery();
con.Close();
return res;
}
public bool IsReusable
{
get
{
return false;
}
}
}
SP
ALTER PROCEDURE dbo.AddHit
(
@url varchar(200)
)
AS
DECLARE @ADID INT
SELECT @ADID = AdID from ADs where
NavigateUrl = @url
IF exists (Select ADID FROM AdHits
where ADID = @ADID)
UPDATE ADHits SET Hits =
Hits+1 where ADID = @ADID
ELSE
INSERT INTO ADHits values
(@ADID,1)
RETURN
This HttpHanlder will accept the ad’s
landing page (or NavigateUrl) as a querystring parameter to record the click
into our database. Once it is recorded, the user will be redirected to the
target ad’s page.
Now, we need to make the AdRotator
control to use this proxy handler to redirect the user instead of passing them
directly.
Well, this can be done by 2
ways.
1. By AdCreated event
The AdCreated event will expose the
advertisements NavigateUrl, ImageUrl, AlternateText properties which can be
changed. You can use this event to pass the user through the proxy handler.
Refer below,
ASPX
<asp:AdRotator ID="AdRotator1"
runat="server" DataSourceID="SqlDataSource1"
onadcreated="AdRotator1_AdCreated" />
<asp:SqlDataSource
ID="SqlDataSource1" runat="server"
ConnectionString="<%$
ConnectionStrings:ConnectionString %>"
SelectCommand="SELECT * FROM
[Ads]"></asp:SqlDataSource>
CodeBehind
protected void AdRotator1_AdCreated(object
sender, AdCreatedEventArgs e)
{
e.NavigateUrl =
"AdHandler.ashx?AdUrl=" + e.NavigateUrl;
}
2. By Ad XML or Database table
When configuring the NavigateUrl
property of the ad, you can use the proxy handler. Something like
below,
<Ad>
<ImageUrl>~/img/AccordionPanel.jpg</ImageUrl>
<NavigateUrl>
AdHandler.ashx?AdUrl=http://www.codedigest.com/Articles/jQuery/335_Creating_Accordion _Panel_using_jQuery_in_ASPNet_%e2%80%93_Part_1.aspx
</NavigateUrl>
<AlternateText>
Creating Accordion Panel using
jQuery in ASP.Net
</AlternateText>
</Ad>
We can now get the report of
hits/clicks of the ads from the ADHits table.
|