Even though, we can implement this simple feature in
Global.asax file we will also learn this feature through HttpModules. By
implementing this simple feature through HttpModules we will understand the
basics of HttpModules and building our own custom HttpModules.
What is an HttpModule?
The HttpModules are the components in worker process
that helps in completing the request given to the server. HttpModules are
similar to ISAPI filters which help the runtime to filter the request given to
the server prior to asp.net release. Developers can implement their own filters
specific to their application needs. These ISAPI filters implementation can be
done through unmanaged code and it is extremely difficult to implement. ISAPI
equivalent in ASP.Net is the HttpModules, which are easy to implement. To
implement this easily, .Netframework has an interface called IHttpModule which
should be utilized with simple config settings.
In ASP.Net, whenever a request is given to the server
(http://localhost/WebApp/Default.aspx), there is a mechanism called HttpPipeline
which happens in the worker process to complete a request given to the server.
HttpPipeline is a mechanism which will receive the request with appropriate
information’s through HttpContext object and passes the request to series of
object for request completion. During this stage, the involvement of HttpModules
comes into picture for servicing the request. There are a number of system-level
(asp.net) HTTP modules, providing services like authentication, state
management, output caching, etc. We can always write our own HttpModules and
configure it for our web application in web.config.
In simple words, writing our own HttpModule will help us
to hook a event in every request processing of our application to implement out
own application specific needs.
To implement a custom HttpModule, we need to implement
IHttpModule interface in System.Web namespace.
public interface IHttpModule
{
// Summary:
// Disposes of the resources (other than
memory) used by the module that implements
// System.Web.IHttpModule.
void Dispose();
//
// Summary:
// Initializes a module and prepares it to
handle requests.
//
// Parameters:
// context:
// An System.Web.HttpApplication that provides
access to the methods, properties,
// and events common to all application objects
within an ASP.NET application
void Init(HttpApplication context);
}
The module that implement the above interface should
implement Init() and Dispose() events. A web.Config entry inside
<System.Web> is needed to hook this module in the request processing.
<httpModules>
<add type="namespace.ClassName, namespace"
name="ClassName" />
</httpModules>
Using a Custom HttpModule
using System;
using System.Collections.Generic;
using System.Text;
using System.Web;
namespace CodeDigestModule
{
public class PageProcessingTime : IHttpModule
{
DateTime dtStart = DateTime.MinValue;
public PageProcessingTime()
{
//
// TODO: Add constructor logic here
//
}
private HttpApplication mApplication;
public void Init(System.Web.HttpApplication
application)
{
// Wire up beginrequest
application.BeginRequest += new
System.EventHandler(BeginRequest);
// Wire up endrequest
application.EndRequest += new
System.EventHandler(EndRequest);
// Save the application
mApplication = application;
}
public void BeginRequest(object sender, EventArgs
e)
{
dtStart = DateTime.Now;
}
public void EndRequest(object sender, EventArgs
e)
{
DateTime dtEnd = DateTime.Now;
TimeSpan tsProcessingTime = dtEnd -
dtStart;
mApplication.Response.Write("<b>Request
Processing Time: Using HttpMoules </b>" +
tsProcessingTime.ToString());
}
public void Dispose()
{
}
}
}
Web.Config
<httpModules>
<add type="CodeDigestModule.PageProcessingTime,
CodeDigestModule" name="PageProcessingTime" />
</httpModules>
When we execute the project, we will get an output with
the processing time at the end of the every page.
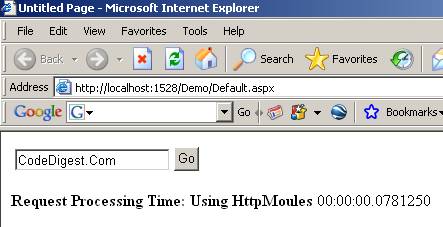
|