In this article, we will implement paging feature with
DataPager control in 3 different ways,
Ø Paging
in ListView through Datasource control databind.
Ø Paging
in ListView without using DataSource Controls.
Ø Providing Custom interface for the Paging in ListView.
Paging in ListView through Datasource
control databind
Before moving to paging implementation
with DataPager we will bind the ListView by configuring a SqlDataSource
control.
Configuring SqlDataSource
Control
Drag a SqlDataSource control from the
data tab of Visual Studio 2008. Configure it with the Database Server; I have
used a database in App_Data folder.
Configuring ListView
Control
Drag a ListView control from the data
tab of Visual Studio 2008. Click the Smart tag of ListView control and select
the datasource control; Refer the below figure, SqlDataSource1 in our example.
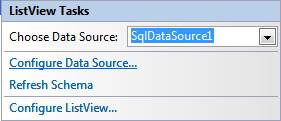
Click “Configure ListView...” option as
seen in the above figure to choose the display Layout for the ListView. Choose a
display layout, I have selected “Flow” in our example, as seen in the below
figure. Click Ok.
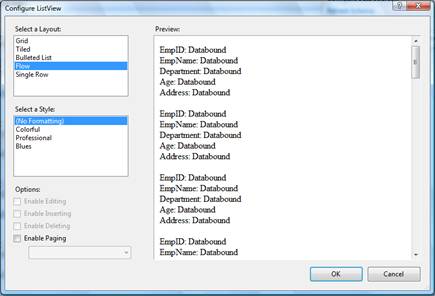
You can check “Enable Paging” in the above figure, which will add a DataPager control in the LayoutTemplate of the ListView control but we will add the DataPager control explicitly in this article. Executing the page will display the
records without paging. Now, we will provide paging feature through the
DataPager control.
Configure Paging through
DataPager
Drag a DataPager control from the data
tab of Visual Studio 2008. Assign the PagedControlID property to the
ListView control’s ID. Set PageSize property if required, default is 10.
I have set as 2 in our example.
There are 2 default pager styles that
can be set to a DataPager control, Next/Previous Pager and Numeric Pager.
Without setting this there will be no pagination displayed for the ListView. To
configure, Click the smart tag of DataPager control and choose the style as
shown in the below figure,
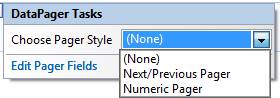
Executing the page will give output
with paging,
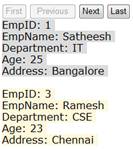
Configuring DataPager inside
ListView
The above walkthrough placed the
DataPager control outside the ListView control, in other words, the paging can
be displayed at any part of the page. In this section, we will see how the
DataPager can be a part of ListView control itself.
It can be done by placing the DataPager
control inside the LayoutTemplate of the ListView. In this case, we neither need
nor set the PagedControlID property because DataPager control itself will
render accordingly when it is placed inside the ListView control.
<asp:ListView ID="ListView1"
runat="server" DataKeyNames="EmpID"
DataSourceID="SqlDataSource1">
<LayoutTemplate>
<asp:DataPager ID="dpListView"
runat="server" PageSize="2">
<Fields>
<asp:NumericPagerField
ButtonType="Button" NextPageText="more.." />
</Fields>
</asp:DataPager>
<div
ID="itemPlaceholderContainer" runat="server"
style="font-family:
Verdana, Arial, Helvetica, sans-serif;">
<span ID="itemPlaceholder"
runat="server" />
</div>
<div style="text-align:
center;background-color: #CCCCCC;font-family: Verdana, Arial, Helvetica,
sans-serif;color: #000000;">
</div>
</LayoutTemplate>
<ItemTemplate>
<span
style="background-color: #DCDCDC;color: #000000;">EmpID:
<asp:Label ID="EmpIDLabel3"
runat="server" Text='<%# Eval("EmpID") %>' />
<br />
EmpName:
<asp:Label
ID="EmpNameLabel1" runat="server" Text='<%# Eval("EmpName") %>'
/>
<br />
Department:
<asp:Label
ID="DepartmentLabel1" runat="server"
Text='<%#
Eval("Department") %>' />
<br />
Age:
<asp:Label ID="AgeLabel1"
runat="server" Text='<%# Eval("Age") %>' />
<br />
Address:
<asp:Label
ID="AddressLabel1" runat="server" Text='<%# Eval("Address") %>'
/>
<br />
<br />
</span>
</ItemTemplate>
</asp:ListView>
In the next section, we will provide
paging to ListView control with DataPager without using DataSource
controls.
Paging with DataPager without using
DataSource Controls
1. Drag a ListView control inside a webform as we did in previous
section.
<asp:ListView ID="lvEmployee" runat="server">
<LayoutTemplate>
<table ID="itemPlaceholderContainer" >
<tr runat="server" ID="itemPlaceholder">
</tr>
</table>
</LayoutTemplate>
<ItemTemplate>
<tr>
<td>
EmpID: <asp:Label ID="EmpIDLabel"
runat="server" Text='<%# Eval("EmpID") %>' />
<br />
EmpName: <asp:Label ID="EmpNameLabel"
runat="server"
Text='<%# Eval("EmpName") %>'
/>
<br />
Department:<asp:Label ID="DepartmentLabel"
runat="server"
Text='<%# Eval("Department") %>' />
<br />
Age: <asp:Label ID="AgeLabel" runat="server"
Text='<%# Eval("Age") %>' />
<br />
Address:<asp:Label ID="AddressLabel"
runat="server" Text='<%# Eval("Address") %>' />
</td>
</tr>
</ItemTemplate>
</asp:ListView>
2. In the codebehind file, assign the DataTable or DataSet to the
DataSource Property of the ListView control like we do for a GridView
control.
protected
void Page_Load(object sender, EventArgs e)
{
if
(!IsPostBack)
{
lvEmployee.DataSource = GetEmployee("Select * from Employee");
lvEmployee.DataBind();
}
}
public
DataTable GetEmployee(string query)
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["DatabaseConnectionString"].ConnectionString);
SqlDataAdapter ada = new SqlDataAdapter(query, con);
DataTable dtEmp = new DataTable();
ada.Fill(dtEmp);
return
dtEmp;
}
3. Drag a DataPager control from the Data tab of visual studio. When we
execute the page, Paging will not work automatically; we need to bind the
ListView to the datasource again in the PreRender event of DataPager.
<asp:DataPager ID="DataPager1" runat="server"
PagedControlID="lvEmployee"
PageSize="2" onprerender="DataPager1_PreRender">
<Fields>
<asp:NextPreviousPagerField ButtonType="Button" />
</Fields>
</asp:DataPager>
protected void
DataPager1_PreRender(object sender, EventArgs e)
{
lvEmployee.DataSource = GetEmployee("Select * from Employee");
lvEmployee.DataBind();
}
Execute the page and you can see your
ListView paging working perfectly.
|